↓Recommended Follow↓
Transformers have fundamentally changed deep learning models since their introduction.
Today, we will unveil the core concepts behind Transformers: the attention mechanism, encoder-decoder architecture, multi-head attention, and more. Through Python code snippets, you’ll gain a deeper understanding of its principles.
1. Understanding the Attention Mechanism
The attention mechanism is a fascinating concept in neural networks, especially for tasks involving NLP. It acts like a spotlight for the model, allowing it to focus on certain parts of the input sequence while ignoring others, just as humans focus on specific words or phrases when understanding a sentence.
Now, let’s delve into a specific type of attention mechanism called self-attention, also known as internal attention. Imagine that when reading a sentence, your brain automatically highlights important words or phrases to grasp the meaning. This is the fundamental principle of self-attention in neural networks. It allows each word in the sequence to “attend” to other words, including itself, to better understand the context.
2. How Self-Attention Works
Here’s how self-attention works in a simple example:
Consider the sentence: “The cat sat on the mat.”
Embedding
First, the model embeds each word in the input sequence into a high-dimensional vector representation. This embedding process allows the model to capture semantic similarities between words.
Query, Key, and Value Vectors
Next, the model computes three vectors for each word in the sequence: the query vector, the key vector, and the value vector. During training, the model learns these vectors, each serving a different purpose. The query vector represents the query of the word, i.e., what the model is looking for in the sequence. The key vector represents the key of the word, which is what other words in the sequence should pay attention to. The value vector represents the value of the word, i.e., the information the word contributes to the output.
Attention Scores
Once the model computes the query, key, and value vectors for each word, it calculates attention scores for each pair of words in the sequence. This is usually done by taking the dot product of the query vector and the key vector to evaluate the similarity between words.
SoftMax Normalization
Then, the attention scores are normalized using the softmax function to obtain attention weights. These weights indicate how much each word should pay attention to other words in the sequence. Words with higher attention weights are considered more critical to the task being performed.
Weighted Sum
Finally, the weighted sum of the value vectors is calculated using the attention weights. This produces the output of the self-attention mechanism for each word in the sequence, capturing contextual information from other words.
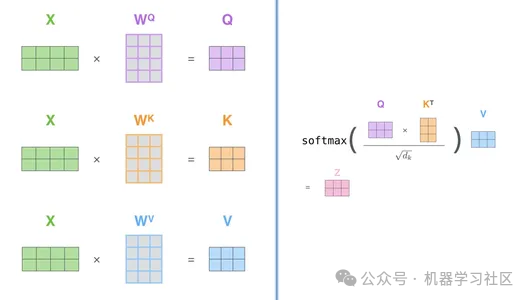
Here’s a simple explanation of computing attention scores:
# Install PyTorch
!pip install torch==2.2.1+cu121
# Import libraries
import torch
import torch.nn.functional as F
# Example input sequence
input_sequence = torch.tensor([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6], [0.7, 0.8, 0.9]])
# Generate random weights for Key, Query, and Value matrices
random_weights_key = torch.randn(input_sequence.size(-1), input_sequence.size(-1))
random_weights_query = torch.randn(input_sequence.size(-1), input_sequence.size(-1))
random_weights_value = torch.randn(input_sequence.size(-1), input_sequence.size(-1))
# Compute Key, Query, and Value matrices
key = torch.matmul(input_sequence, random_weights_key)
query = torch.matmul(input_sequence, random_weights_query)
value = torch.matmul(input_sequence, random_weights_value)
# Compute attention scores
attention_scores = torch.matmul(query, key.T) / torch.sqrt(torch.tensor(query.size(-1), dtype=torch.float32))
# Use softmax function to obtain attention weights
attention_weights = F.softmax(attention_scores, dim=-1)
# Compute weighted sum of Value vectors
output = torch.matmul(attention_weights, value)
print("Output after self-attention:")
print(output)
3. Basics of the Transformer Model
Before we delve into the complex workings of the Transformer model, let’s take a moment to appreciate its groundbreaking architecture. As discussed earlier, the Transformer model has reshaped the landscape of natural language processing (NLP) by introducing a novel approach centered around the self-attention mechanism. In the following sections, we will uncover the core components of the Transformer model, elucidating its encoder-decoder architecture, positional encoding, multi-head attention, and feed-forward networks.
Encoder-Decoder Architecture
At the heart of the Transformer is its encoder-decoder architecture—a symbiotic relationship between two key components responsible for processing input sequences and generating output sequences. Each layer in both the encoder and decoder contains the same sub-layers, including self-attention mechanisms and feed-forward networks. This architecture not only aids in comprehensively understanding the input sequence but also generates context-rich output sequences.
Positional Encoding
While the Transformer model is powerful, it lacks an intrinsic understanding of the order of elements—a limitation addressed by positional encoding. By combining input embeddings with positional information, positional encoding allows the model to distinguish the relative positions of elements in the sequence. This nuanced understanding is crucial for capturing the temporal dynamics of language and facilitating accurate comprehension.
Multi-Head Attention
A notable feature of the Transformer model is its ability to simultaneously attend to different parts of the input sequence—achieved through multi-head attention. By splitting the query, key, and value vectors into multiple heads and performing independent self-attention computations, the model gains a nuanced perspective on the input sequence, enriching its representation with diverse contextual information.
Feed-Forward Networks
Similar to the human brain’s ability to process information in parallel, each layer in the Transformer model contains a feed-forward network—a versatile component that captures complex relationships between elements in the sequence. By using linear transformations and non-linear activation functions, the feed-forward network enables the model to navigate the complex semantic landscape of language, facilitating robust understanding and generation of text.
4. Detailed Explanation of Transformer Components
To implement, first run the code for positional encoding, multi-head attention mechanism, and feed-forward networks, followed by the encoder, decoder, and Transformer architecture.
# Import libraries
import math
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
1. Positional Encoding
In the Transformer model, positional encoding is a key component that injects information about token positions into the input embeddings.
Unlike recurrent neural networks (RNNs) or convolutional neural networks (CNNs), Transformers lack intrinsic knowledge of token positions due to their permutation invariance. Positional encoding addresses this limitation by providing the model with positional information, allowing it to process sequences in the correct order.
Concept of Positional Encoding
Typically, positional encoding is added to the input embeddings before passing them into the Transformer model. It consists of a set of sine functions with varying frequencies and phases, allowing the model to distinguish tokens based on their position in the sequence.
The formula for positional encoding is as follows
Assuming you have an input sequence of length L and need to find the position of the k-th object in that sequence. The positional encoding is given by sine and cosine functions of different frequencies:
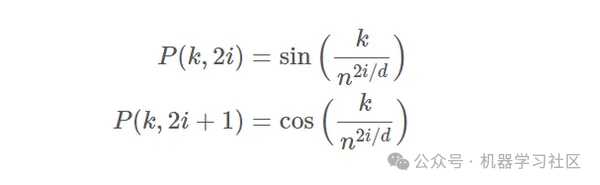
Where:
-
k: The position of the object in the input sequence, 0≤k<L/2 -
d: The dimension of the output embedding space -
P(k,j): The position function that maps the position k in the input sequence to the index (k,j) in the position matrix -
n: A user-defined scalar set to 10,000 by the authors of “Attention Is All You Need”. -
i: The value used to map column indices to 0≤i<d/2, with a single i value mapping to both sine and cosine functions.
Different Positional Encoding Schemes
Various positional encoding schemes are used in Transformers, each with its advantages and disadvantages:
-
Fixed Positional Encoding: In this scheme, positional encodings are predefined and remain fixed for all sequences. While simple and efficient, fixed positional encodings may fail to capture complex patterns in sequences. -
Learned Positional Encoding: Another option is to learn positional encodings during training, allowing the model to adaptively capture positional information from the data. Learned positional encodings offer greater flexibility but require more parameters and computational resources.
Implementation of Positional Encoding
Let’s implement positional encoding in Python:
# Implementation of Positional Encoding
class PositionalEncoding(nn.Module):
def __init__(self, d_model, max_len=5000):
super(PositionalEncoding, self).__init__()
# Calculate positional encoding
pe = torch.zeros(max_len, d_model)
position = torch.arange(0, max_len, dtype=torch.float).unsqueeze(1)
div_term = torch.exp(torch.arange(0, d_model, 2).float() * (-torch.log(
torch.tensor(10000.0)) / d_model))
pe[:, 0::2] = torch.sin(position * div_term)
pe[:, 1::2] = torch.cos(position * div_term)
pe = pe.unsqueeze(0)
self.register_buffer('pe', pe)
def forward(self, x):
x = x + x + self.pe[:, :x.size(1)]
return x
# Example usage
d_model = 512
max_len = 100
num_heads = 8
# Positional Encoding
pos_encoder = PositionalEncoding(d_model, max_len)
# Example input sequence
input_sequence = torch.randn(5, max_len, d_model)
# Apply positional encoding
input_sequence = pos_encoder(input_sequence)
print("Shape of input sequence after positional encoding:")
print(input_sequence.shape)
2. Multi-Head Attention Mechanism
In the Transformer architecture, the multi-head attention mechanism is a key component that enables the model to simultaneously focus on different parts of the input sequence. It allows the model to capture complex dependencies and associations within the sequence, improving performance on tasks such as language translation, text generation, and sentiment analysis.
Importance of Multi-Head Attention
Multi-head attention has several advantages:
-
Parallelization: By simultaneously focusing on different parts of the input sequence, multi-head attention significantly speeds up computation, making it more efficient than traditional attention mechanisms. -
Enhanced Representation: Each attention head focuses on different aspects of the input sequence, allowing the model to capture various patterns and relationships. This leads to richer and more powerful representations of the input, enhancing the model’s ability to understand and generate text. -
Improved Generalization: Multi-head attention enables the model to attend to both local and global dependencies within the sequence, thereby improving generalization across different tasks and domains.
Computation of Multi-Head Attention:
Let’s break down the steps involved in computing multi-head attention:
-
Linear Transformations: The input sequence undergoes learnable linear transformations, projecting it into multiple lower-dimensional representations called “heads.” Each head focuses on different aspects of the input, enabling the model to capture various patterns. -
Scaled Dot-Product Attention: Each head independently computes attention scores between the query, key, and value representations of the input sequence. This step involves calculating the similarity between tokens and their context, scaled by the square root of the model depth. The resulting attention weights highlight the importance of each token relative to others. -
Concatenation and Linear Projection: The attention outputs from all heads are concatenated and linearly projected back to the original dimension. This process combines insights from multiple heads, enhancing the model’s ability to understand complex relationships within the sequence.
Code Implementation
Let’s translate the theory into code:
# Code implementation of Multi-Head Attention
class MultiHeadAttention(nn.Module):
def __init__(self, d_model, num_heads):
super(MultiHeadAttention, self).__init__()
self.num_heads = num_heads
self.d_model = d_model
assert d_model % num_heads == 0
self.depth = d_model // num_heads
# Linear projections for Query, Key, and Value
self.query_linear = nn.Linear(d_model, d_model)
self.key_linear = nn.Linear(d_model, d_model)
self.value_linear = nn.Linear(d_model, d_model)
# Output linear projection
self.output_linear = nn.Linear(d_model, d_model)
def split_heads(self, x):
batch_size, seq_length, d_model = x.size()
return x.view(batch_size, seq_length, self.num_heads, self.depth).transpose(1, 2)
def forward(self, query, key, value, mask=None):
# Linear projections
query = self.query_linear(query)
key = self.key_linear(key)
value = self.value_linear(value)
# Split heads
query = self.split_heads(query)
key = self.split_heads(key)
value = self.split_heads(value)
# Scaled dot-product attention
scores = torch.matmul(query, key.transpose(-2, -1)) / math.sqrt(self.depth)
# Apply mask if provided
if mask is not None:
scores += scores.masked_fill(mask == 0, -1e9)
# Calculate attention weights and apply softmax
attention_weights = torch.softmax(scores, dim=-1)
# Apply attention to values
attention_output = torch.matmul(attention_weights, value)
# Merge heads
batch_size, _, seq_length, d_k = attention_output.size()
attention_output = attention_output.transpose(1, 2).contiguous().view(batch_size,
seq_length, self.d_model)
# Linear projection
attention_output = self.output_linear(attention_output)
return attention_output
# Example usage
d_model = 512
max_len = 100
num_heads = 8
d_multihead_attn = MultiHeadAttention(d_model, num_heads)
# Example input sequence
input_sequence = torch.randn(5, max_len, d_model)
# Multi-head attention
attention_output= multihead_attn(input_sequence, input_sequence, input_sequence)
print("Shape of attention output:", attention_output.shape)
3. Feed-Forward Networks
In the context of Transformers, feed-forward networks play a critical role in processing information and extracting features from the input sequence. They are the backbone of the model, facilitating the transformation of representations between different layers.
Role of Feed-Forward Networks
The feed-forward networks within each Transformer layer are responsible for applying non-linear transformations to the input representations. They allow the model to capture complex patterns and relationships in the data, facilitating the learning of higher-level features.
Structure and Function of Feed-Forward Layers
The feed-forward layer consists of two linear transformations separated by a non-linear activation function (typically ReLU). Let’s break down the structure and function:
-
Linear Transformation 1: Projects the input representation into a higher-dimensional space using a learnable weight matrix. -
Non-Linear Activation: The output from the first linear transformation is passed through a non-linear activation function (e.g., ReLU). This introduces non-linearity into the model, enabling it to capture complex patterns and relationships in the data. -
Linear Transformation 2: The output of the activation function is then projected back to the original dimensional space through another learnable weight matrix.
Code Implementation
Let’s implement the feed-forward network in Python:
# Code implementation of Feed-Forward Network
class FeedForward(nn.Module):
def __init__(self, d_model, d_ff):
super(FeedForward, self).__init__()
self.linear1 = nn.Linear(d_model, d_ff)
self.linear2 = nn.Linear(d_ff, d_model)
self.relu = nn.ReLU()
def forward(self, x):
# Linear Transformation 1
x = self.relu(self.linear1(x))
# Linear Transformation 2
x = self.linear2(x)
return x
# Example usage
d_model = 512
max_len = 100
num_heads = 8
d_ff = 2048
# Multi-head attention
multihead_attn = MultiHeadAttention(d_model, num_heads)
# Feed-forward network
ff_network = FeedForward(d_model, d_ff)
# Example input sequence
input_sequence = torch.randn(5, max_len, d_model)
# Multi-head attention
attention_output= multihead_attn(input_sequence, input_sequence, input_sequence)
# Feed-forward network
output_ff = ff_network(attention_output)
print('input_sequence',input_sequence.shape)
print("output_ff", output_ff.shape)
4. The Encoder
Plays a crucial role in the Transformer model, primarily tasked with converting the input sequence into meaningful representations, capturing important information from the input.
Structure and Function of Each Encoder Layer
The encoder consists of multiple layers, each containing the following components in sequence: input embeddings, positional encoding, multi-head self-attention mechanism, and position-wise feed-forward networks.
-
Input Embedding: We first convert the input sequence into a dense vector representation called input embeddings. We use pre-trained word embeddings or embeddings learned during training to map each word in the input sequence into a high-dimensional vector space.
-
Positional Encoding: We add positional encoding to the input embeddings to incorporate the sequential information of the input sequence. This enables the model to distinguish the positions of words in the sequence, overcoming the lack of sequential information in traditional neural networks.
-
Multi-Head Self-Attention Mechanism: After positional encoding, the input embeddings pass through a multi-head self-attention mechanism. This mechanism allows the encoder to weigh the importance of different words in the input sequence based on their relationships. By focusing on relevant parts of the input sequence, the encoder can capture long-range dependencies and semantic relationships.
-
Position-Wise Feed-Forward Network: Following the self-attention mechanism, the encoder applies a position-wise feed-forward network independently to each position. This network consists of two linear transformations separated by a non-linear activation function (typically ReLU). It helps capture complex patterns and relationships in the input sequence.
Code Implementation
Let’s take a look at the code for implementing an encoder layer with input embeddings and positional encoding in Python:
# Code implementation of Encoder
class EncoderLayer(nn.Module):
def __init__(self, d_model, num_heads, d_ff, dropout):
super(EncoderLayer, self).__init__()
self.self_attention = MultiHeadAttention(d_model, num_heads)
self.feed_forward = FeedForward(d_model, d_ff)
self.norm1 = nn.LayerNorm(d_model)
self.norm2 = nn.LayerNorm(d_model)
self.dropout = nn.Dropout(dropout)
def forward(self, x, mask):
# Self-attention layer
attention_output= self.self_attention(x, x,
x, mask)
attention_output = self.dropout(attention_output)
x = x + attention_output
x = self.norm1(x)
# Feed-forward layer
feed_forward_output = self.feed_forward(x)
feed_forward_output = self.dropout(feed_forward_output)
x = x + feed_forward_output
x = self.norm2(x)
return x
d_model = 512
max_len = 100
num_heads = 8
d_ff = 2048
# Multi-head attention
encoder_layer = EncoderLayer(d_model, num_heads, d_ff, 0.1)
# Example input sequence
input_sequence = torch.randn(1, max_len, d_model)
# Multi-head attention
encoder_output= encoder_layer(input_sequence, None)
print("encoder output shape:", encoder_output.shape)
5. The Decoder
Plays a vital role in the Transformer model, generating the output sequence based on the encoded representation of the input sequence. It receives the encoded input sequence from the encoder and uses it to generate the final output sequence.
Function of the Decoder
The primary function of the decoder is to generate the output sequence while attending to relevant parts of the input sequence and previously generated tokens. It utilizes the encoded representation of the input sequence to understand the context and make informed decisions about generating the next token.
Components of the Decoder Layer
The decoder layer includes the following components:
-
Output Embedding Shifted Right: Before processing the input sequence, the model shifts the output embeddings one position to the right. This ensures that each token in the decoder can receive the correct context from previously generated tokens during training.
-
Positional Encoding: Similar to the encoder, the model adds positional encoding to the output embeddings to incorporate the sequential information of the tokens. This encoding helps the decoder distinguish tokens based on their position in the sequence.
-
Masked Multi-Head Self-Attention Mechanism: The decoder employs a masked multi-head self-attention mechanism to attend to relevant parts of the input sequence and previously generated tokens. During training, the model applies a mask to prevent attention to future tokens, ensuring that each token can only attend to previous tokens.
-
Encoder-Decoder Attention Mechanism: In addition to the masked self-attention mechanism, the decoder also includes an encoder-decoder attention mechanism. This mechanism allows the decoder to attend to relevant parts of the input sequence, aiding in generating output tokens influenced by the input context.
-
Position-Wise Feed-Forward Network: After the attention mechanism, the decoder applies a position-wise feed-forward network independently to each token. This network captures complex patterns and relationships in the input and previously generated tokens, helping to generate accurate output sequences.
Code Implementation
# Code implementation of Decoder
class DecoderLayer(nn.Module):
def __init__(self, d_model, num_heads, d_ff, dropout):
super(DecoderLayer, self).__init__()
self.masked_self_attention = MultiHeadAttention(d_model, num_heads)
self.enc_dec_attention = MultiHeadAttention(d_model, num_heads)
self.feed_forward = FeedForward(d_model, d_ff)
self.norm1 = nn.LayerNorm(d_model)
self.norm2 = nn.LayerNorm(d_model)
self.norm3 = nn.LayerNorm(d_model)
self.dropout = nn.Dropout(dropout)
def forward(self, x, encoder_output, src_mask, tgt_mask):
# Masked self-attention layer
self_attention_output= self.masked_self_attention(x, x, x, tgt_mask)
self_attention_output = self.dropout(self_attention_output)
x = x + self_attention_output
x = self.norm1(x)
# Encoder-decoder attention layer
enc_dec_attention_output= self.enc_dec_attention(x, encoder_output,
encoder_output, src_mask)
enc_dec_attention_output = self.dropout(enc_dec_attention_output)
x = x + enc_dec_attention_output
x = self.norm2(x)
# Feed-forward layer
feed_forward_output = self.feed_forward(x)
feed_forward_output = self.dropout(feed_forward_output)
x = x + feed_forward_output
x = self.norm3(x)
return x
# Define parameters for DecoderLayer
d_model = 512 # Dimension of the model
num_heads = 8 # Number of attention heads
d_ff = 2048 # Dimension of the feed-forward network
dropout = 0.1 # Dropout probability
batch_size = 1 # Batch size
max_len = 100 # Maximum length of the sequence
# Define an instance of DecoderLayer
decoder_layer = DecoderLayer(d_model, num_heads, d_ff, dropout)
src_mask = torch.rand(batch_size, max_len, max_len) > 0.5
tgt_mask = torch.tril(torch.ones(max_len, max_len)).unsqueeze(0) == 0
# Pass the input tensor to DecoderLayer
output = decoder_layer(input_sequence, encoder_output, src_mask, tgt_mask)
# Output shape
print("Output shape:", output.shape)
5. Transformer Model Architecture
The synthesis of the various components discussed in the previous sections. Let’s gather the knowledge of the encoder, decoder, attention mechanisms, positional encoding, and feed-forward networks to understand how the complete Transformer model is constructed and operates.
Overview of the Transformer Model
At its core, the Transformer model consists of stacked encoder and decoder modules designed to process input sequences and generate output sequences. Here’s a high-level overview of the architecture:
Encoder
-
The encoder module processes the input sequence, extracting features and creating rich representations of the input. -
It consists of multiple encoder layers, each containing self-attention mechanisms and feed-forward networks. -
The self-attention mechanism allows the model to simultaneously focus on different parts of the input sequence, capturing dependencies and associations. -
We add positional encoding to the input embeddings to provide information about the positions of tokens in the sequence.
Decoder
-
The decoder module takes the output of the encoder as input and generates the output sequence. -
Similar to the encoder, it consists of multiple decoder layers, each containing self-attention, encoder-decoder attention, and feed-forward networks. -
In addition to self-attention, the decoder also includes encoder-decoder attention to focus on the input sequence while generating output. -
As with the encoder, we add positional encoding to the input embeddings to provide positional information.
Connections and Normalization
-
Between each layer of the encoder and decoder modules, there are residual connections followed by layer normalization. -
These mechanisms help gradients flow through the network and stabilize training.
The complete Transformer model is constructed by stacking multiple encoder and decoder layers. Each layer processes the input sequence independently, allowing the model to learn hierarchical representations and capture complex patterns in the data. The encoder passes its output to the decoder, which generates the final output sequence based on the input.
Implementation of the Transformer Model
Let’s implement the complete Transformer model in Python:
# Implementation of the TRANSFORMER
class Transformer(nn.Module):
def __init__(self, src_vocab_size, tgt_vocab_size, d_model, num_heads, num_layers, d_ff,
max_len, dropout):
super(Transformer, self).__init__()
# Define embedding layers for encoder and decoder
self.encoder_embedding = nn.Embedding(src_vocab_size, d_model)
self.decoder_embedding = nn.Embedding(tgt_vocab_size, d_model)
# Define positional encoding layer
self.positional_encoding = PositionalEncoding(d_model, max_len)
# Define stacked layers for encoder and decoder
self.encoder_layers = nn.ModuleList([EncoderLayer(d_model, num_heads, d_ff, dropout)
for _ in range(num_layers)])
self.decoder_layers = nn.ModuleList([DecoderLayer(d_model, num_heads, d_ff, dropout)
for _ in range(num_layers)])
# Define linear layer
self.linear = nn.Linear(d_model, tgt_vocab_size)
self.dropout = nn.Dropout(dropout)
# Generate masks
def generate_mask(self, src, tgt):
src_mask = (src != 0).unsqueeze(1).unsqueeze(2)
tgt_mask = (tgt != 0).unsqueeze(1).unsqueeze(3)
seq_length = tgt.size(1)
nopeak_mask = (1 - torch.triu(torch.ones(1, seq_length, seq_length), diagonal=1)).bool()
tgt_mask = tgt_mask & nopeak_mask
return src_mask, tgt_mask
# Forward propagation
def forward(self, src, tgt):
src_mask, tgt_mask = self.generate_mask(src, tgt)
# Word embeddings and positional encoding for encoder input
encoder_embedding = self.encoder_embedding(src)
en_positional_encoding = self.positional_encoding(encoder_embedding)
src_embedded = self.dropout(en_positional_encoding)
# Word embeddings and positional encoding for decoder input
decoder_embedding = self.decoder_embedding(tgt)
de_positional_encoding = self.positional_encoding(decoder_embedding)
tgt_embedded = self.dropout(de_positional_encoding)
enc_output = src_embedded
for enc_layer in self.encoder_layers:
enc_output = enc_layer(enc_output, src_mask)
dec_output = tgt_embedded
for dec_layer in self.decoder_layers:
dec_output = dec_layer(dec_output, enc_output, src_mask, tgt_mask)
output = self.linear(dec_output)
return output
# Example usage
src_vocab_size = 5000
tgt_vocab_size = 5000
d_model = 512
num_heads = 8
num_layers = 6
d_ff = 2048
max_len = 100
dropout = 0.1
transformer = Transformer(src_vocab_size, tgt_vocab_size, d_model, num_heads, num_layers,
d_ff, max_len, dropout)
# Generate random example data
src_data = torch.randint(1, src_vocab_size, (5, max_len)) # (batch_size, seq_length)
tgt_data = torch.randint(1, tgt_vocab_size, (5, max_len)) # (batch_size, seq_length)
transformer(src_data, tgt_data[:, :-1]).shape
6. Training and Evaluation of the Model
Training a Transformer model involves optimizing its parameters to minimize a loss function, typically using gradient descent and backpropagation. Once training is complete, various metrics are used to evaluate the model’s performance in solving the target tasks.
Training Process
Gradient Descent and Backpropagation:
-
During training, the input sequence is fed into the model, generating an output sequence. -
The model’s predictions are compared with the ground truth, involving the use of a loss function (e.g., cross-entropy loss) to measure the difference between predicted and actual values. -
Gradient descent is used to update the model’s parameters in the direction that minimizes the loss. -
The optimizer adjusts the parameters based on these gradients, iteratively updating them to improve model performance.
Learning Rate Scheduling:
-
Learning rate scheduling techniques can be applied to dynamically adjust the learning rate during training. -
Common strategies include warm-up schedules, where the learning rate starts low and gradually increases, and decay schedules, where the learning rate decreases over time.
Evaluation Metrics
Perplexity:
-
Perplexity is a common metric used to evaluate the performance of language models, including Transformers. -
It measures the model’s ability to predict a given sequence of tokens. -
Lower perplexity values indicate better performance, ideally approaching the size of the vocabulary.
BLEU Score:
-
BLEU (Bilingual Evaluation Understudy) score is commonly used to assess the quality of machine-translated text. -
It compares generated translations to one or more reference translations provided by human translators. -
BLEU scores range from 0 to 1, with higher scores indicating better translation quality.
7. Implementation of Training and Evaluation
Let’s look at the basic code implementation for training and evaluating the Transformer model using PyTorch:
# Training and evaluation of the Transformer model
criterion = nn.CrossEntropyLoss(ignore_index=0)
optimizer = optim.Adam(transformer.parameters(), lr=0.0001, betas=(0.9, 0.98), eps=1e-9)
# Training loop
transformer.train()
for epoch in range(10):
optimizer.zero_grad()
output = transformer(src_data, tgt_data[:, :-1])
loss = criterion(output.contiguous().view(-1, tgt_vocab_size), tgt_data[:, 1:]
.contiguous().view(-1))
loss.backward()
optimizer.step()
print(f"Epoch {epoch+1}: Loss= {loss.item():.4f}")
# Dummy data
src_data = torch.randint(1, src_vocab_size, (5, max_len)) # (batch_size, seq_length)
tgt_data = torch.randint(1, tgt_vocab_size, (5, max_len)) # (batch_size, seq_length)
# Evaluation loop
transformer.eval()
with torch.no_grad():
output = transformer(src_data, tgt_data[:, :-1])
loss = criterion(output.contiguous().view(-1, tgt_vocab_size), tgt_data[:, 1:]
.contiguous().view(-1))
print(f"\nEvaluation loss on dummy data= {loss.item():.4f}")
8. Advanced Topics and Applications
Transformers have sparked a multitude of advanced concepts and applications in the field of natural language processing (NLP). Let’s delve into some of these topics, including different variants of attention, BERT (Bidirectional Encoder Representations from Transformers), and GPT (Generative Pre-trained Transformer), along with their practical applications.
Different Variants of Attention
The attention mechanism is central to the Transformer model, allowing it to focus on relevant parts of the input sequence. Various variants of attention have been proposed to enhance the capabilities of Transformers.
-
Scaled Dot-Product Attention: The standard attention mechanism used in the original Transformer model. It takes the dot product of the query and key vectors as the attention scores, scaled by the square root of the dimension.
-
Multi-Head Attention: A powerful extension of attention that utilizes multiple attention heads to simultaneously capture different aspects of the input sequence. Each head learns different attention patterns, allowing the model to focus on various parts of the input in parallel.
-
Relative Positional Encoding: Introduces relative positional encoding to capture the relative positional relationships between tokens more effectively. This variant enhances the model’s ability to understand the sequential relationships between tokens.
BERT (Bidirectional Encoder Representations from Transformers)
BERT is a landmark Transformer-based model that has had a profound impact on NLP. It is pre-trained on a large-scale text corpus using objectives such as masked language modeling and next sentence prediction. BERT learns deep contextual representations of words, capturing bidirectional context, which enables it to perform well across a wide range of downstream NLP tasks.
Code snippet – BERT model:
from transformers import BertModel, BertTokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
inputs = tokenizer("Hello, world!", return_tensors="pt")
outputs = model(**inputs)
print(outputs)
GPT (Generative Pre-trained Transformer)
GPT is a Transformer-based model known for its generative capabilities. Unlike BERT, which is bidirectional, GPT adopts a decoder-only architecture and autoregressive training to generate coherent and contextually relevant text. Researchers and developers have successfully applied GPT to various tasks, such as text completion, summarization, and dialogue generation.
Code snippet – GPT model:
from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
model = GPT2LMHeadModel.from_pretrained('gpt2')
input_text = "Once upon a time, "
inputs=tokenizer(input_text,return_tensors='pt')
output=tokenizer.decode(
model.generate(
**inputs,
max_new_tokens=100,
)[0],
skip_special_tokens=True
)
input_ids = tokenizer(input_text, return_tensors='pt')
print(output)
9. Conclusion
Transformers have revolutionized the field of natural language processing (NLP) with their ability to capture context and understand language.
Through attention mechanisms, encoder-decoder architectures, and multi-head attention, they have enabled tasks such as machine translation and sentiment analysis to be performed at an unprecedented scale. As we continue to explore models like BERT and GPT, it is clear that Transformers are at the forefront of language understanding and generation.
Their impact on NLP is profound, and the journey of discovery alongside Transformers will unveil even more remarkable advancements in the field.
Research Papers
-
“Attention is All You Need” -
“BERT: Pre-training of Deep Bidirectional Transformers for Language Understanding” -
“Language Models are Unsupervised Multitask Learners” -
Attention in transformers, visually explained -
Transformer Neural Networks, ChatGPT’s foundation
(End)
AI Algorithm Exchange Group (Knowledge Planet) has arrived, a technical communication and job exchange platform for all students and machine learning/algorithm engineers/researchers.
Here you can learn about cutting-edge AI technology news, papers, large models (LLM), algorithm competitions, practical projects, get AI algorithm campus recruitment/job search preparation strategies, interview question banks, interview experience sharing, offer selection, internal referral opportunities, learning routes, job search Q&A and a wealth of learning materials and more.
At the same time, you can interact with developers from prestigious universities and companies such as HKUST, Peking University, Tsinghua University, Chinese Academy of Sciences, CMU, Tencent, Baidu, etc.~
The AI algorithm exchange group covers multiple directions including search advertising, deep learning, machine learning, computer vision, knowledge graphs, natural language processing, big data, autonomous driving, robotics, large models (including ChatGPT) and more.
We will periodically carry out cash discount activities for Knowledge Planet, before joining the planet you can add my WeChat: mlc2060, to inquire about the activity details. iOS users can directly add my WeChat to join the planet(Planet coin payment is not supported)