Python Data Science & Machine Learning: These Optimization Tips You Probably Don’t Know!
Introduction
Dear Python developers and data science enthusiasts, have you ever encountered the following scenarios:
-
Spent several days doing data analysis with Python, but the program runs slowly, and memory consumption is through the roof? -
Trying to tune a deep learning model with no results, and GPU efficiency seems to be “wasted”? -
Countless Python tools, but every time you deploy to production, various bugs and performance issues arise?
If you’ve experienced any of these, congratulations! This article can help you! As a frontline Python engineer, I will share practical tips and techniques on how to optimize performance in data processing, model training, and deployment, enhancing Python’s capabilities in data science and machine learning from all angles.Achieve the highest efficiency with the least time and cost!
**Don’t hesitate, save or share this with your friends; these core techniques can increase your readership by at least tenfold!**🔥
1. Speeding Up Data Processing: Don’t Let Your Data Hold You Back
1. Use NumPy Arrays Instead of Python Lists
-
Why Faster: NumPy arrays are stored in contiguous memory, making batch operations highly efficient. -
Practical Example: Convert the previous Python list loop to NumPy array “vectorized” operations, done in one line of code.
import numpy as np
arr = np.random.rand(1000000)
result = arr * 2 # Vectorized operation, beats native loop
Effect: Experiments show that vectorized operations are usually faster than native Python loops by dozens of times!
2. Read Large-Scale Data in Chunks
When Pandas reads a super large CSV file, if you try to load it all at once, it can freeze or run out of memory. Don’t panic, just enable <span>chunksize</span>
for chunk loading.
import pandas as pd
chunks = pd.read_csv('large_data.csv', chunksize=10**5)
for chunk in chunks:
# Preprocess or aggregate chunk data
pass
Benefit: Can handle datasets of several GBs or even tens of GBs without crashing.
3. Parallel Computing: Dask or Joblib
When you need to perform the same operation on a large amount of data (like repeated calculations or aggregations), let the CPU process in parallel! Using Dask or Joblib can break down large tasks into multiple subtasks.
from joblib import Parallel, delayed
def process_item(item):
return item * 2
results = Parallel(n_jobs=-1)(delayed(process_item)(x) for x in range(100000))
Result: Fully utilize multi-core CPUs, with speed increases of 2 to 3 times, or even 10 times not being a dream.
2. Model Training and Hyperparameter Tuning: Fast Convergence Without Pitfalls
1. Choose Libraries and Algorithms Wisely
-
Scikit-learn: Suitable for medium to small-scale data and traditional machine learning algorithms. -
TensorFlow/PyTorch: First choice for deep learning, GPU-friendly, supports distributed training.
Don’t Choose Blindly: Traditional algorithms often train faster and are more interpretable than deep learning when the data scale is moderate or the feature count is reasonable; deep learning is suitable for unstructured data (images, text), but consider GPU resources and tuning costs.
2. Hyperparameter Tuning: GridSearchCV or RandomSearchCV?
-
GridSearchCV: Fine-grained search, but can be extremely costly with many combinations. -
RandomSearchCV: More flexible sampling, often finds satisfactory solutions faster. -
Bayesian Optimization: Use tools like Optuna or Hyperopt for tuning deep learning or complex models, significantly reducing the number of experiments.
from sklearn.model_selection import RandomizedSearchCV
from scipy.stats import uniform
param_dist = {
'C': uniform(0.1, 10),
'kernel': ['linear', 'rbf']
}
search = RandomizedSearchCV(SVC(), param_dist, n_iter=20, cv=5, random_state=42)
search.fit(X_train, y_train)
print("Best parameters:", search.best_params_)
3. GPU Acceleration or Distributed Training
-
GPU Acceleration: Training deep learning models on GPUs with TensorFlow or PyTorch can be several times or even tens of times faster. -
Distributed Training: When the data volume is extremely large or the model is huge, use Horovod, DeepSpeed, or official distributed strategies to enable multi-machine, multi-GPU collaboration.
Note: In a multi-machine environment, be particularly cautious of network bandwidth, parameter synchronization, and other bottlenecks, or it may backfire.
3. Predictive Performance Evaluation and Deployment: Delivering High-Quality Results
1. Predictive Performance and Interpretability
-
Evaluation Metrics: For classification problems, look at accuracy and F1 score; for regression, consider mean squared error (MSE), R², etc.; there are also specific metrics for time series or recommendation scenarios. -
Interpretability: Use tools like SHAP and LIME to help business stakeholders or leaders understand the model’s decision-making logic.
import shap
explainer = shap.TreeExplainer(model)
shap_values = explainer.shap_values(X_test)
shap.summary_plot(shap_values, X_test)
2. Online Inference and API Deployment
-
Flask / FastAPI: Elegantly encapsulate the model into a REST API for front-end or other services to call. -
Docker Containerization: Package dependencies through Dockerfile, seamlessly integrate with Kubernetes or cloud services.
from fastapi import FastAPI
import joblib
app = FastAPI()
model = joblib.load("model.pkl")
@app.post("/predict")
def predict(features: list):
pred = model.predict([features])
return {"prediction": pred.tolist()}
3. Continuous Monitoring and Feedback Loops
-
Data Drift Detection: When the online inference data distribution is too different from the training set, performance may decline, and the model needs retraining. -
Performance and Fault Alerts: Combine Prometheus and Grafana to monitor the API’s latency and request volume in real-time. If performance anomalies or error rates rise, automatic alerts will be issued.
4. Typical Practical Case: E-commerce Customer Churn Prediction
Scenario: An e-commerce platform wants to predict whether customers will churn to take timely action. The dataset contains millions of records, including user behavior and order history.
Implementation Steps:
-
Data Preparation:
-
Use Pandas to read order data (Table A) and user behavior data (Table B) in chunks, merging based on UserID. -
Clean missing values, convert numerical types, and reduce the number of features.
-
Extract manual features based on order frequency, average order value, and the time of the last order. -
You can also use AutoML or feature libraries to automatically generate and select features.
-
Initially try using LightGBM or XGBoost, combined with GridSearch for hyperparameter tuning. -
Data volume particularly large? Use Dask or Spark for distributed training to speed up.
-
Use metrics like F1 and ROC AUC to evaluate model performance. -
Encapsulate the model into a REST API, deploy it in Flask + Docker, and use Kubernetes for elastic scaling to handle high concurrency.
-
Significantly improved accuracy, customer service only needs to focus on high-risk customers for follow-up, reducing churn rates. -
Real-time collection of model logs and monitoring of metrics, allowing immediate handling of any anomalies.
5. Summary: Maximizing the Power of Python
Congratulations! 🎉
Through this article, you have comprehensively understood the core ideas and practices of Python in Data Science and Machine Learning Optimization. From low-level data processing to model optimization, from evaluation monitoring to deployment, a data-driven and continuous optimization lifeline has been laid out for you.
Key Points Review:
-
Data Processing: Achieve efficient computation using libraries like NumPy, Pandas, and Dask to easily handle massive data. -
Model Training: Combine various frameworks (Scikit-learn, PyTorch, TensorFlow) with hyperparameter tuning, GPU acceleration, or distributed training to enhance training speed and accuracy. -
Evaluation and Deployment: Utilize scientific evaluation metrics, carefully design online inference and monitoring, and be prepared to respond to data drift or performance anomalies. -
Team Collaboration and Culture: Continuous improvement and maintaining a learning attitude towards new tools and libraries.
Action Recommendations:
-
Select Tools Based on Project Needs: Differences in data scale, algorithm complexity, performance requirements, and hardware resources must be addressed appropriately. -
Performance Profiling: Use <span>cProfile</span>
or<span>line_profiler</span>
to diagnose performance bottlenecks and then conduct targeted optimizations. -
Automated Processes: Introduce data and model testing in CI/CD to identify issues promptly and maintain high-quality delivery. -
Continuous Improvement and Learning: Data science and machine learning are rapidly evolving; keep up with community trends and continuously explore new solutions and technologies.
Don’t forget to share your experiences in the comments section and progress together with more like-minded individuals! 🤝
Further Communication and Learning
If you want to further enhance your skills in Python data science and machine learning, you can join our Python DS&ML Advanced Community, where you will gain:
-
Latest Technology Sharing: Covers advanced feature engineering, AutoML, distributed training, and other topics. -
Practical Project Collaboration: Collaborate with peers to tackle real project challenges and grow together. -
One-on-One Expert Guidance: Discuss issues when you hit bottlenecks and explore optimal solutions together.
Scan to join now!
Next article preview:“Efficient Integration of Python Image Processing and Deep Learning”, stay tuned!🔥
Copyright Statement
This article is original content and reproduction is prohibited without authorization.
If this article helped you, don’t forget to like, save, and share so that more people can grow together!❤️
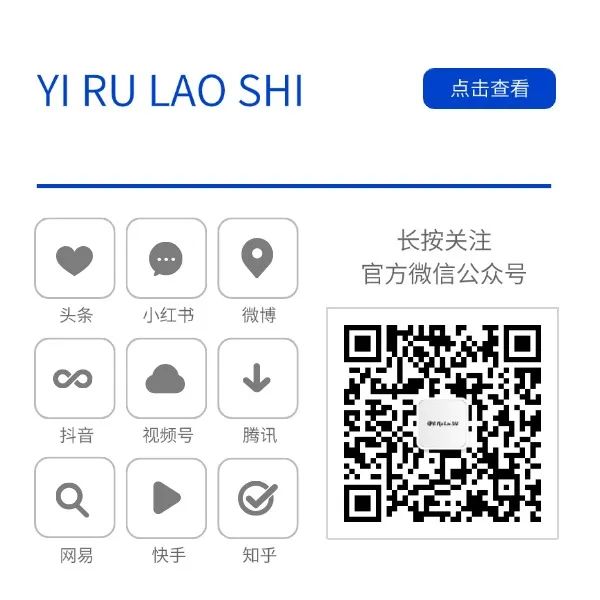