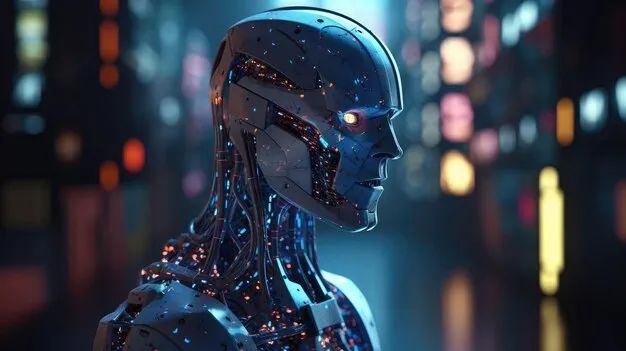
Chatbot development is a challenging and complex task that requires a combination of various technologies and tools. In this field, the combination of LLAMA, LangChain, and Python forms a powerful trio, providing excellent support for the design and implementation of chatbots.
First, LLAMA is a powerful natural language processing tool with advanced semantic understanding and dialogue management capabilities. It helps chatbots better understand user intent and respond intelligently based on context. The high customizability of LLAMA allows developers to flexibly adjust the language processing capabilities of the chatbot according to actual needs.
LangChain, as a full-stack language technology platform, provides rich development resources for chatbots. It integrates multiple language technologies, including speech recognition, text processing, and machine translation, offering comprehensive support for multimodal interactions. The powerful features of LangChain enable developers to easily build complex and flexible chatbot systems.
Python, as a general-purpose programming language, is an ideal choice for chatbot development. Its simple yet powerful syntax makes the development process more efficient, while its rich ecosystem of third-party libraries provides a wide array of tools and resources for chatbot development. The cross-platform nature of Python also allows chatbots to run in different environments, achieving broader applications.
Chatbot development relies heavily on large language models (LLMs), which are language models that have garnered attention for their ability to achieve general language understanding and generation capabilities. LLMs learn billions of parameters during training by using vast amounts of data and consume substantial computational resources during training and operation to acquire these capabilities.
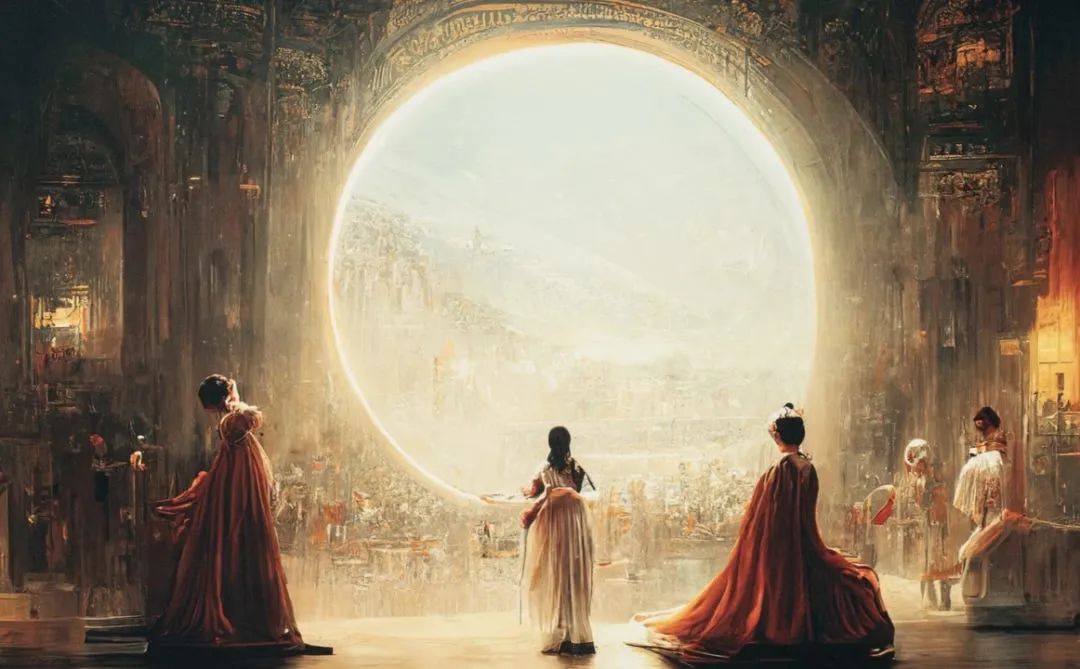
Let’s build a simple chatbot using LangChain, LLAMA, and Python!
In this simple project, I want to create a chatbot focused on a specific topic related to HIV/AIDS. This means that the messages sent to the chatbot will be answered based on the association between the topic and the messages. But before that, we need to install and download some necessary components:
I am using LLAMA 2 from META AI, downloaded from Hugging Face.
A framework for developing applications powered by language models.
pip install langchain
3. Install Llama-cpp-python
The Python implementation of the llama.cpp library (I tried using the latest version of llama.cpp, but it didn’t work, so I recommend using the stable version 0.1.78 and ensure that a C++ compiler is installed).
pip install llama-cpp-python==0.1.78
from langchain.prompts import PromptTemplate
from langchain.llms import LlamaCpp
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import (
StreamingStdOutCallbackHandler
)
PromptTemplate: Responsible for creating PromptValue, which is an object that dynamically combines values based on user input.
LlamaCpp: The C/C++ port of Facebook’s LLAMA model.
CallbackManager: Handles callbacks from LangChain.
StreamingStdOutCallbackHandler: A callback handler for streaming.
Code
First, I will create a variable called <span>"your_model_path"</span>
for my model path, and since I want to limit the topic to HIV/AIDS, I will create a topic variable called <span>"chat_topic"</span>
and fill it with <span>"HIV/AIDS"</span>
. Obviously, you can modify this topic, and if you do not want to limit the topic, you can remove <span>"chat_topic"</span>
and change the template. After that, I will create a variable called <span>"user_question"</span>
to receive user input, along with a template that will be used later.
your_model_path = "write your model path"
chat_topic = "hiv/aids"
user_question = str(input("Enter your question: "))
template= """
Please explain this question: "{question}", the topic is about {topic}
"""
I will create a variable for the <span>PromptTemplate</span>
that will use the template we created earlier and assign it to the <span>"prompt"</span>
variable, then change the format of the prompt and assign it to the <span>"final_prompt"</span>
variable. We use the topic from <span>"chat_topic"</span>
and the question we initialized in <span>"user_question"</span>
. Then create a variable called <span>"CallbackManager"</span>
and assign the streaming handler to it.
prompt = PromptTemplate.from_template(template)
final_prompt = prompt.format(
topic=chat_topic,
question=user_question
)
CallbackManager = CallbackManager([StreamingStdOutCallbackHandler()])
Next, let’s create the model.
llm = LlamaCpp(
model_path=your_model_path,
n_ctx=6000,
n_gpu_layers=512,
n_batch=30,
callback_manager=CallbackManager,
temperature=0.9,
max_tokens=4095,
n_parts=1,
verbose=0
)
<span>model_path</span>
: The path of the LLAMA model.
<span>n_ctx</span>
: The token context window, the number of tokens the model can accept when generating a response.
<span>n_gpu_layers</span>
: The number of layers to load into GPU memory.
<span>n_batch</span>
: The number of tokens processed in parallel.
<span>callback_manager</span>
: Handles callbacks.
<span>temperature</span>
: The temperature used for sampling; a higher temperature leads to more creative and imaginative text, while a lower temperature leads to more accurate and practical text.
<span>max_tokens</span>
: The maximum number of tokens generated.
<span>n_parts</span>
: The number of parts to split the model into.
<span>verbose</span>
: Print detailed output.
Finally, call the model and pass the prompt.
python "your_filename.py"
To run it, just type the above command in the cmd.

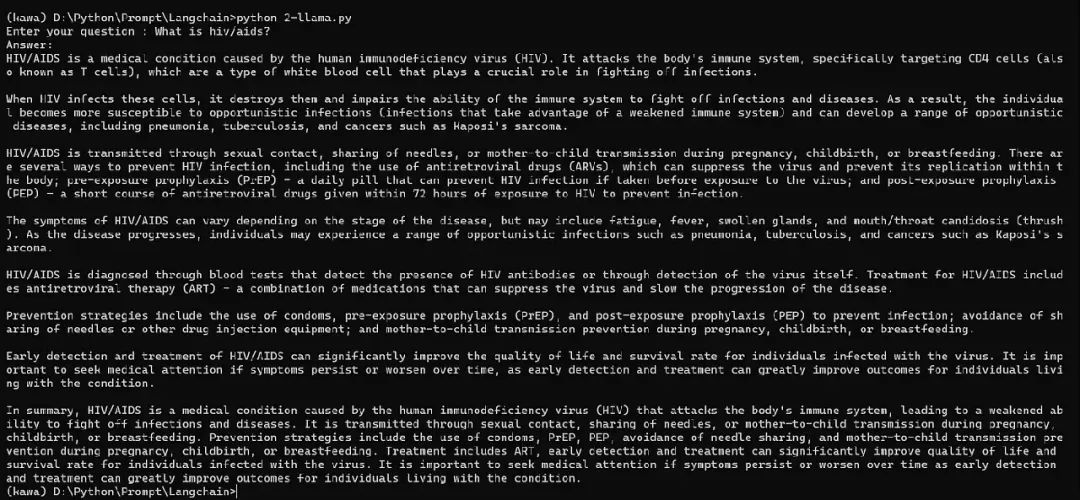
from langchain.prompts import PromptTemplate
from langchain.llms import LlamaCpp
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import (
StreamingStdOutCallbackHandler
)
your_model_path = "write your model path"
chat_topic = "hiv/aids"
user_question = str(input("Enter your question: "))
template= """
Please explain this question: "{question}", the topic is about {topic}
"""
prompt = PromptTemplate.from_template(template)
final_prompt = prompt.format(
topic=chat_topic,
question=user_question
)
CallbackManager = CallbackManager([StreamingStdOutCallbackHandler()])
llm = LlamaCpp(
model_path=your_model_path,
n_ctx=6000,
n_gpu_layers=512,
n_batch=30,
callback_manager=CallbackManager,
temperature=0.9,
max_tokens=4095,
n_parts=1,
verbose=0
)
llm(final_prompt)