Utilize LangChain, LangGraph, and Yahoo Finance to build a stock performance analysis agent.
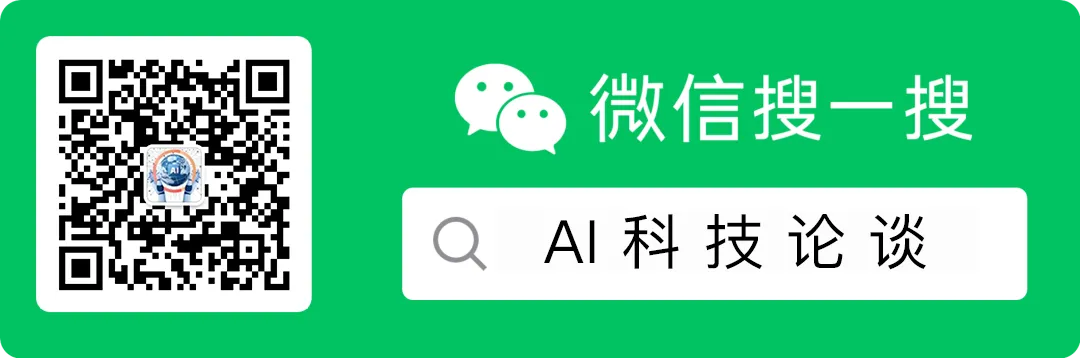
AI Empowered Stock Analysis
In stock trading, investors rely on various tools and methods to make informed decisions. One method is fundamental analysis, which provides operational advice by evaluating a company’s financials against stock performance. With the development of AI and machine learning, stock analysis can now be largely automated.
This tutorial introduces how to build a stock performance analysis agent using LangChain, LangGraph, and Yahoo Finance, utilizing real-time data and key performance indicators. Let’s get started!
Features of the Financial Analysis Agent
-
Obtain stock price data using Yahoo Finance. -
Calculate technical indicators such as Relative Strength Index (RSI), Moving Average Convergence Divergence (MACD), and Volume Weighted Average Price (VWAP). -
Evaluate financial metrics like Price-to-Earnings ratio, Debt-to-Equity ratio, and Profit Margin. -
Utilize OpenAI’s powerful language model to provide structured AI analysis results.
Required Tools
-
LangGraph: A library for orchestrating tools and building conversational agents. -
OpenAI GPT-4: For generating intelligent and structured financial insights. -
yfinance: For obtaining stock prices and financial ratios. -
ta (Technical Analysis Library): For calculating key technical indicators. -
Python libraries (pandas, dotenv, datetime): For data processing and environment setup.
Step 1: Environment Setup
First, install the required libraries:
pip install -U langgraph langchain langchain_openai pandas ta python-dotenv yfinance
Next, set up a<span>.env</span>
file to securely store your OpenAI API key:
OPENAI_API_KEY=your OpenAI API key
Step 2: Analysis Tools
Get Stock Prices
This tool is designed to fetch historical data for stocks and calculate multiple technical indicators.
from typing import Union, Dict, Set, List, TypedDict, Annotated
import pandas as pd
from langchain_core.tools import tool
import yfinance as yf
from ta.momentum import RSIIndicator, StochasticOscillator
from ta.trend import SMAIndicator, EMAIndicator, MACD
from ta.volume import volume_weighted_average_price
@tool
def get_stock_prices(ticker: str) -> Union[Dict, str]:
"""Fetch historical stock price data and technical indicators for a given ticker"""
try:
data = yf.download(
ticker,
start=dt.datetime.now() - dt.timedelta(weeks=24*3),
end=dt.datetime.now(),
interval='1wk'
)
df= data.copy()
data.reset_index(inplace=True)
data.Date = data.Date.astype(str)
indicators = {}
rsi_series = RSIIndicator(df['Close'], window=14).rsi().iloc[-12:]
indicators["RSI"] = {date.strftime('%Y-%m-%d'): int(value)
for date, value in rsi_series.dropna().to_dict().items()}
sto_series = StochasticOscillator(
df['High'], df['Low'], df['Close'], window=14).stoch().iloc[-12:]
indicators["Stochastic_Oscillator"] = {
date.strftime('%Y-%m-%d'): int(value)
for date, value in sto_series.dropna().to_dict().items()}
macd = MACD(df['Close'])
macd_series = macd.macd().iloc[-12:]
indicators["MACD"] = {date.strftime('%Y-%m-%d'): int(value)
for date, value in macd_series.to_dict().items()}
macd_signal_series = macd.macd_signal().iloc[-12:]
indicators["MACD_Signal"] = {date.strftime('%Y-%m-%d'): int(value)
for date, value in macd_signal_series.to_dict().items()}
vwap_series = volume_weighted_average_price(
high=df['High'], low=df['Low'], close=df['Close'],
volume=df['Volume'],
).iloc[-12:]
indicators["vwap"] = {date.strftime('%Y-%m-%d'): int(value)
for date, value in vwap_series.to_dict().items()}
return {'stock_price': data.to_dict(orient='records'),
'indicators': indicators}
except Exception as e:
return f"Error fetching price data: {str(e)}"
Financial Ratios
This tool fetches key financial health ratios.
@tool
def get_financial_metrics(ticker: str) -> Union[Dict, str]:
"""Fetch key financial ratios for a given ticker"""
try:
stock = yf.Ticker(ticker)
info = stock.info
return {
'pe_ratio': info.get('forwardPE'),
'price_to_book': info.get('priceToBook'),
'debt_to_equity': info.get('debtToEquity'),
'profit_margins': info.get('profitMargins')
}
except Exception as e:
return f"Error fetching ratios: {str(e)}"
Step 3: Build LangGraph
LangGraph allows us to effectively orchestrate tools and manage conversational logic.
1. Define the Graph
We first define a StateGraph to manage the workflow:
from langgraph.graph import StateGraph, START, END
class State(TypedDict):
messages: Annotated[list, add_messages]
stock: str
graph_builder = StateGraph(State)
2. Define OpenAI and Bind Tools
Integrate the tools into LangGraph and create an analysis feedback loop.
import dotenv
dotenv.load_dotenv()
from langchain_openai import ChatOpenAI
llm = ChatOpenAI(model='gpt-4o-mini')
tools = [get_stock_prices, get_financial_metrics]
llm_with_tool = llm.bind_tools(tools)
3. Analyst Node
The following prompt is designed to allow the AI to understand its role and provide structured output.
FUNDAMENTAL_ANALYST_PROMPT = """
You are a fundamental analyst focused on evaluating the performance of companies (ticker: {company}) based on stock prices, technical indicators, and financial metrics. Your task is to provide a comprehensive summary of fundamental analysis for the given stock.
You can use the following tools:
1. **get_stock_prices**: Fetch the latest stock prices, historical price data, and technical indicators such as RSI, MACD, and VWAP.
2. **get_financial_metrics**: Fetch key financial metrics such as revenue, earnings per share (EPS), price-to-earnings (P/E) ratio, and debt-to-equity ratio.
### Your Task:
1. **Input Stock Ticker**: Use the provided stock ticker to query the tools and collect relevant information.
2. **Analyze Data**: Evaluate the results from the tools and identify potential resistance, key trends, strengths, or points of concern.
3. **Provide Summary**: Write a concise and well-structured summary highlighting the following:
- Recent stock price trends, patterns, and potential resistance.
- Key insights from technical indicators (e.g., whether the stock is overbought or oversold).
- Financial health and performance based on financial metrics.
### Constraints:
- Only use data provided by the tools.
- Avoid speculative language; focus on observable data and trends.
- If any tool fails to provide data, clearly state that in the summary.
### Output Format:
Respond in the following format:
"stock": "<ticker>",
"price_analysis": "<detailed analysis of stock price trends>",
"technical_analysis": "<detailed time series analysis of all technical indicators>",
"financial_analysis": "<detailed analysis of financial metrics>",
"final Summary": "<complete conclusions based on the above analyses>",
"Asked Question Answer": "<answers based on the above details and analysis>"
Make sure your response is objective, concise, and actionable.
"""
def fundamental_analyst(state: State):
messages = [
SystemMessage(content=FUNDAMENTAL_ANALYST_PROMPT.format(company=state['stock'])),
] + state['messages']
return {
'messages': llm_with_tool.invoke(messages)
}
graph_builder.add_node('fundamental_analyst', fundamental_analyst)
graph_builder.add_edge(START, 'fundamental_analyst')
4. Adding Tools and Compiling the Graph
graph_builder.add_node(ToolNode(tools))
graph_builder.add_conditional_edges('fundamental_analyst', tools_condition)
graph_builder.add_edge('tools', 'fundamental_analyst')
graph = graph_builder.compile()
5. Execute the Graph
events = graph.stream({'messages':[('user', 'Should I buy this stock?')],
'stock': 'TSLA'}, stream_mode='values')
for event in events:
if 'messages' in event:
event['messages'][-1].pretty_print()
Example Output
{
"stock": "TSLA",
"price_analysis": "Tesla (TSLA) has shown significant volatility in recent weeks, with a sharp decline from a high of $361.53 on November 18, 2024, to a low of $147.05 on April 15, 2024. The current stock price is approximately $352.56, indicating a strong rebound from the recent low. The potential resistance appears to be around $360 based on recent highs, while support can be identified around $320.",
"technical_analysis": "Technical indicators present a mixed outlook. The RSI recently rose to 71, indicating the stock is nearing the overbought region. The Stochastic Oscillator shows a value of 94, also suggesting the stock may be overbought. Meanwhile, the MACD has been rising, currently at 28, indicating upward momentum. However, caution is advised due to the potential overbought signals from both the RSI and Stochastic Oscillator.",
"financial_analysis": "Tesla's financial metrics indicate a high valuation relative to earnings, with a P/E ratio of 108.09 and a price-to-book ratio of 16.17. The company's debt-to-equity ratio is 18.08, relatively low, suggesting a strong balance sheet. The profit margin is 13.08%, indicating reasonable profitability. However, the high P/E ratio suggests that investors have high expectations for future growth.",
"final Summary": "In summary, Tesla's stock has rebounded strongly in recent weeks, with potential resistance around $360. Technical indicators suggest the stock may be overbought, which could lead to a price correction. Financially, while the company is performing well with manageable debt levels and reasonable profit margins, the high valuation metrics indicate that future performance must meet elevated expectations. Investors should carefully weigh these factors before deciding to purchase.",
"Asked Question Answer": "Given the current overbought indicators and high valuation, it may be wise to consider waiting for a potential pullback before purchasing Tesla."
}
Future Directions for Improvement
Integrating a portfolio management agent into this project would be a great enhancement. Collaboration among multiple expert teams can strengthen the agent, covering more areas and providing a more comprehensive portfolio management tool.
Building a financial analysis agent not only benefits learning AI and financial analysis but also serves as a foundation for creating powerful practical applications. Try it yourself and experience the power of automation in real-world operations!
Recommended Reading List
“Development and Application Practice of Financial Large Models”
“Development and Application Practice of Financial Large Models” provides a step-by-step, in-depth explanation of the core knowledge of financial large model development and application practice, and demonstrates the usage of various knowledge points through specific examples. The book consists of 11 chapters, covering foundational concepts of large models, data preprocessing and feature engineering, financial time series analysis, financial risk modeling and management, high-frequency trading and quantitative trading, asset pricing and trading strategy optimization, financial market sentiment analysis, blockchain and financial technology innovation, quantitative trading systems based on deep reinforcement learning (OpenAI Baselines + FinRL + DRL + PyPortfolioOpt), futures trading systems based on trend-following (Technical Analysis library + yfinance + Quantstats), and valuation systems for listed companies (OpenAI + LangChain + Tableau + PowerBI). “Development and Application Practice of Financial Large Models” is easy to read, introducing complex cases with minimal text while also covering historical references that are rarely addressed in similar books, making it an ideal tutorial for learning financial large model development.
Purchase Link: https://item.jd.com/14335703.html
Highlights
AI programming assistant Cline releases version 3.1, aiming to replace Cursor and Windsurf.
In 2025, 20 noteworthy RAG frameworks to watch, some open-source (Part 2).
In 2025, 20 noteworthy RAG frameworks to watch, some open-source (Part 1).
In 2025, 10 AI technology trends to watch.
Using LangChain, CrewAI, and AutoGen to build data analysis agents.
IBM launches document processing tool Docling, creating RAG applications based on LangChain.
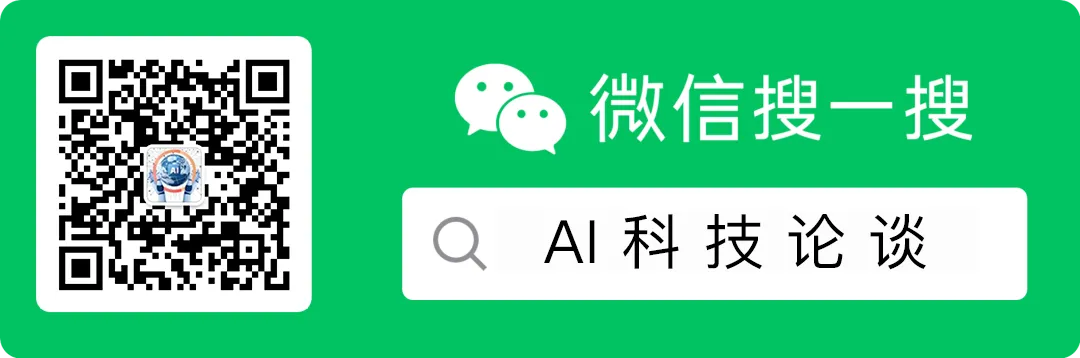
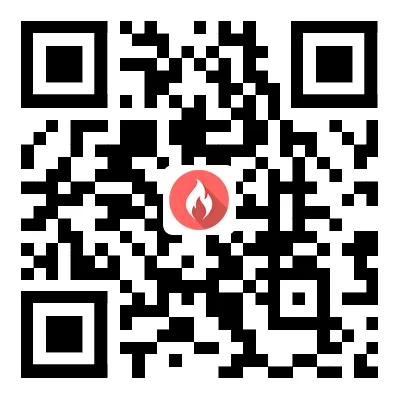