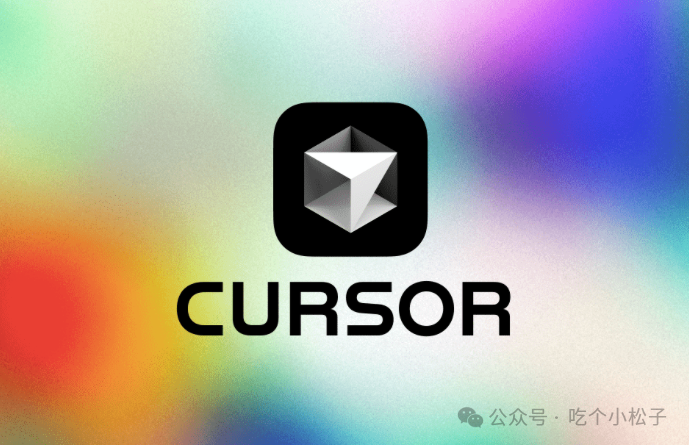
Image classification sounds profound? Don’t worry! With Cursor, this AI programming tool, not only can we save our hair, but we can also code like the wind. Today, I’ll take you on a journey to create an automated image recognition project that is simple and fun. No need to thank me.
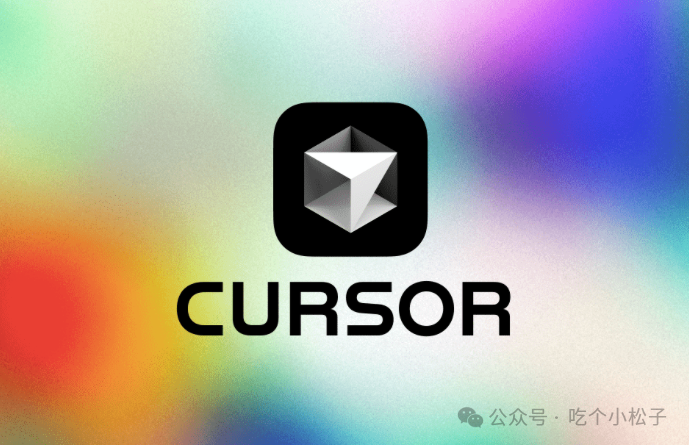
What is Cursor? What can it help us with?
Cursor is an AI programming assistant focused on transforming your coding experience from “never-ending” to “nothing left to write”. Whether it’s code completion, debugging, or logic optimization, Cursor acts like your reliable programmer buddy behind the scenes. Using it for image recognition feels like having a high-energy TA on standby 24/7.
What are we going to do?
This time, our goal is to create an image classifier that can automatically identify whether an image contains a cat or a dog (or something else). With Python and Cursor’s assistance, we can achieve this effortlessly.
Let’s Code Together! From Zero to Image Classification Tool
Preparation Work
First, we need to set up the environment and install some essential tools. We will use <span>TensorFlow</span>
and <span>Keras</span>
, and we need some image data. Cursor will help you with code completion, making it incredibly convenient.
pip install tensorflow keras matplotlib
Once these are installed, we’re good to go. Then, open Cursor and create a new project, naming the file <span>image_classifier.py</span>
.
Step One: Data Preparation
Find some image data from Kaggle or any open-source library. For instance, there’s a famous cat and dog classification dataset that works well. Organize the data into folders for cats and dogs and place them in the project directory.
Cursor can help you write code to automatically load the data, like this:
import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Data preprocessing
datagen = ImageDataGenerator(
rescale=1.0 / 255,
validation_split=0.2
)
train_data = datagen.flow_from_directory(
'data/',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='training'
)
val_data = datagen.flow_from_directory(
'data/',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='validation'
)
Don’t worry about making mistakes, Cursor will give you prompts and help complete the code. Doesn’t it feel like cheating?
Step Two: Build the Model
Next, we need to create a neural network. Let’s go for a simple Convolutional Neural Network (CNN), which is sufficient. Cursor will assist you with code completion and explain why we write it this way.
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(150, 150, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
model.compile(
optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy']
)
Step Three: Train the Model
With the data and model ready, we can get started. Using the code generated by Cursor, your model can easily run:
history = model.fit(
train_data,
validation_data=val_data,
epochs=10
)
Cursor will even remind you to adjust parameters like the <span>epochs</span>
value. After running, you can have it help you plot the training curve to see how the model performs.
import matplotlib.pyplot as plt
# Plot accuracy curve
plt.plot(history.history['accuracy'], label='Train Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.legend()
plt.show()
Isn’t it super easy?
Step Four: Test the Model
Once training is complete, we should test how well the model can classify new images. Cursor will help you write the code and explain each step.
import numpy as np
from tensorflow.keras.preprocessing import image
# Load test image
img = image.load_img('test_image.jpg', target_size=(150, 150))
img_array = image.img_to_array(img) / 255.0
img_array = np.expand_dims(img_array, axis=0)
# Prediction
prediction = model.predict(img_array)
if prediction[0] > 0.5:
print("It's a dog!")
else:
print("It's a cat!")
Upload an image, run this code, and you’ll get the answer in no time.
Final Part: Cursor’s Magic
In this task, Cursor’s main contributions are:
-
Fast coding: precise completion, easy debugging. -
Quick error correction: try intentionally making mistakes and see how smart it is. -
Fast learning: you can learn a lot from its suggestions.
So, have you learned something new? If you still don’t understand, don’t rush, feel free to leave a message! Keep going, the joy of coding is endless!