
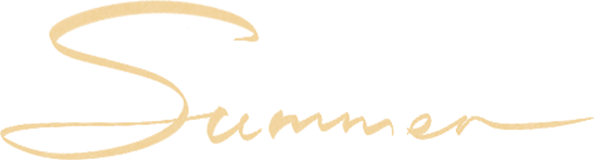
This article introduces how to use Python and the PhiData library to build a simple AI agent. This agent can interact with a simulated environment (including temperature and humidity), and make decisions based on real-time sensor data.
Key points
Define a simple environment class, simulating changes in temperature and humidity.
Create an AI agent class, implementing decision logic using PhiData.
Train the agent to operate in the environment, and log its actions and state transitions.
Optional step: Use Matplotlib to visualize the agent’s performance.
PhiData provides a high-level abstraction layer, simplifying the development of agent-based systems.
This content was generated by AI summary

In this article, we will explore how to use PhiData library to build an AI agent in Python. PhiData is a powerful Python library designed to create and manage complex workflows, especially suitable for building AI agents, automating tasks, and integrating machine learning models. While PhiData is typically used for data processing and handling data pipelines, it can also be extended to model reinforcement learning agents or automate decision-making processes.
We will guide you through the installation and setup of PhiData, implementing AI agents, and the key steps to utilize the functionalities provided by PhiData for task automation or data analysis.
What is PhiData?
PhiData is a Python library that simplifies the creation of data-driven workflows and agent-based systems. It abstracts many low-level operations and provides developers with a higher-level API to create intelligent agents capable of executing complex tasks. Although PhiData is commonly used for data analysis and automating business workflows, it can also be applied to implement AI agents with decision-making capabilities.
The agent model in PhiData is particularly suitable for:
- Data-driven task automation .
- Workflow optimization .
- Decision-making in dynamic environments .
In this tutorial, we will create a simple AI agent that interacts with the environment, makes decisions, and performs actions based on certain data using PhiData.
Step 1 : Install PhiData library
Before we start coding, you need to install PhiData library. As of the writing of this article, PhiData can be obtained via pip.
Install PhiData via pip:
pip install phidata
Additionally, if you need to use NumPy or Matplotlib libraries for numerical or visualization tasks, make sure to install them as well.
pip install numpy matplotlib
Step 2 : Set up the environment
To create an AI agent using PhiData, we first need to set up a custom environment that our agent will interact with. For simplicity, let’s simulate an environment where the agent will make decisions based on sensor data.
In this example, we assume the environment involves tracking temperature and humidity levels and deciding whether to activate a fan or a heater based on these values.
We will:
- Define the environment (temperature and humidity).
- Set up the agent logic using PhiData to make decisions based on data.
Create the Environment class
import random
import numpy as np
class SimpleEnvironment: def __init__(self): self.temperature = 20 # Initial temperature in Celsius self.humidity = 50 # Initial humidity percentage self.time_step = 0 def get_state(self): """Return the current state of the environment.""" return np.array([self.temperature, self.humidity]) def update_state(self): """Simulate state changes (temperature and humidity fluctuations).""" self.temperature += random.uniform(-0.5, 0.5) # Random fluctuation self.humidity += random.uniform(-1, 1) # Random fluctuation self.time_step += 1 def reset(self): """Reset environment to its initial state.""" self.temperature = 20 self.humidity = 50 self.time_step = 0
Explanation of SimpleEnvironment:
- The environment simulates two variables:temperature and humidity .
- The methodget_state() returns the current state of the environment, which the AI agent will use to make decisions.
- The methodupdate_state() simulates random changes in temperature and humidity over time.
- The methodreset() allows resetting the environment to its initial state.
Step 3 : Create AI agent
Next, we will create an AI agent that interacts with the environment. The agent will observe the state of the environment and decide whether to turn on the fan or heater based on predefined thresholds.
We will model the agent’s decision logic using PhiData to define tasks and automate the decision-making process.
Create the Agent class
import phidata as pd
class SimpleAgent: def __init__(self, environment): self.environment = environment self.action_space = ['turn_on_fan', 'turn_on_heater', 'do_nothing'] # Define a task in PhiData self.task = pd.Task( name="Temperature and Humidity Control", input_vars=["temperature", "humidity"], output_vars=["action"], function=self.decide_action ) def decide_action(self, inputs): """Decide the best action based on the current state of the environment.""" temperature, humidity = inputs['temperature'], inputs['humidity'] # Simple decision-making logic if temperature > 25: action = 'turn_on_fan' # Turn on the fan if it's too hot elif temperature < 18: action = 'turn_on_heater' # Turn on the heater if it's too cold else: action = 'do_nothing' # No action needed if temperature is comfortable return {"action": action} def act(self): """Use the PhiData task to decide on an action.""" state = self.environment.get_state() inputs = {"temperature": state[0], "humidity": state[1]} # Execute the task to decide the action result = self.task.execute(inputs) action = result["action"] return action def train(self, steps=100): """Run the agent in the environment for a specified number of steps.""" for step in range(steps): # Make a decision action = self.act() # Perform the action if action == 'turn_on_fan': print("Fan turned on.") elif action == 'turn_on_heater': print("Heater turned on.") else: print("No action taken.") # Update the environment state self.environment.update_state() # Optionally: Add logic for reward or further learning # Reset environment at intervals if needed if step % 50 == 0: self.environment.reset() print("Environment reset.")# Initialize the environmentenv = SimpleEnvironment()# Create the agentagent = SimpleAgent(environment=env)# Train the agent for 100 stepsagent.train(steps=100)
Explanation of the SimpleAgent class:
- Initialization : Initializes the agent using the environment and a predefined action space (three actions: turn on the fan, turn on the heater, or do nothing).
- Task : The agent’s decision-making logic is encapsulated in a PhiData task. This task takes the current state of the environment (temperature and humidity) as input and outputs the action that the agent will take.
- Action selection : The agent’s decide_action method determines whether to turn on the fan, the heater, or do nothing based on simple rules.
- Training : The train() method runs the agent through a series of steps where it makes decisions, performs actions, updates the environment, and repeats. This method can be extended for more complex training processes.
PhiData task:
- Task execution : The task function is executed by PhiData, which handles interactions with the environment state and provides outputs for the agent (the action it should take).
Step 4 : Visualize results (optional)
We can also track the agent’s performance over time by logging its actions and state transitions. Here’s how to visualize the agent’s actions and state transitions using Matplotlib.
import matplotlib.pyplot as plt
class VisualizeAgentPerformance: def __init__(self): self.actions_taken = [] self.temperature_history = [] self.humidity_history = [] def log(self, action, temperature, humidity): self.actions_taken.append(action) self.temperature_history.append(temperature) self.humidity_history.append(humidity) def plot(self): plt.figure(figsize=(12, 6)) plt.subplot(2, 1, 1) plt.plot(self.temperature_history, label="Temperature (°C)") plt.plot(self.humidity_history, label="Humidity (%)") plt.legend() plt.title("Environment State Over Time") plt.subplot(2, 1, 2) plt.plot(self.actions_taken, label="Actions Taken", linestyle='--') plt.yticks([0, 1, 2], ['Do Nothing', 'Turn on Fan', 'Turn on Heater']) plt.title("Agent Actions Over Time") plt.show()# Initialize the visualizervisualizer = VisualizeAgentPerformance()# Training with loggingfor step in range(100): action = agent.act() state = env.get_state() visualizer.log(action, state[0], state[1]) env.update_state()visualizer.plot()
Conclusion
In this article, we built a simple AI agent using PhiData library in Python. The agent interacts with a simulated environment (temperature and humidity control) and makes decisions based on real-time sensor data. We walked through the process of defining the environment, creating the agent, implementing decision logic using PhiData tasks, and visualizing the agent’s performance over time.
PhiData provides a higher level of abstraction for agent-based systems, simplifying the development of intelligent agents by focusing on data-driven task automation and decision workflows. You can extend this framework to more complex environments, such as robotic control systems, task automation in business processes, or real-time decision systems.
PhiData flexibility and powerful capabilities make it an ideal tool for quickly prototyping AI agents that need to handle and process complex data streams.
Related Recommendations
21 Most Detailed Guides to RAG Framework Before 2025, Including Features, Applicable Scenarios, Advantages, and Core Functions
A Comprehensive Introduction to AI Agents for Developers, Key Attributes, and Delegation Capabilities Between Agents
Creating Compelling Product Advertisements Using Generative AI Models
The Role of Generative AI in Automating Customer Service Tasks
Good News for Self-Media: Use this Tool to Generate 175,314 Images with AI in Two Months
Human Intelligence Exchange
Bringing Future Intelligence Closer
Facilitating Communication Platform Between Humans and Future Intelligence
All Network | Human Intelligence Exchange
Join the Communication Group ·
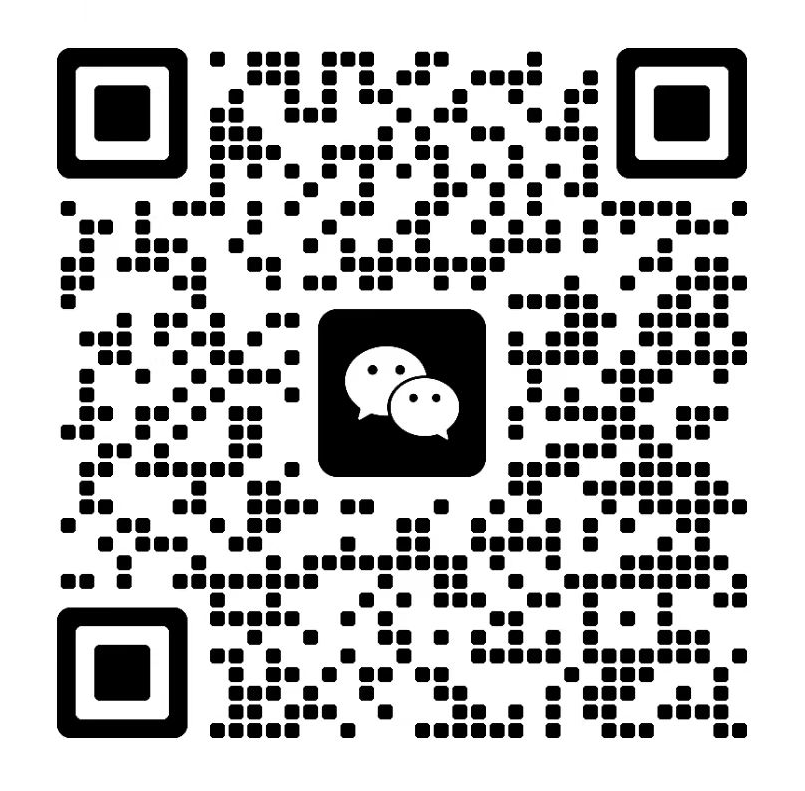
![]() |
AIGC Communication Group · |
![]() |
Office Applications Communication Group |
![]() |
AI Programmer Communication Group |