
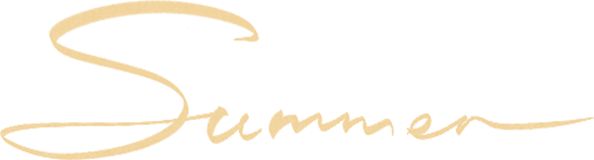
This article introduces how to use Python, PhiData, FastAPI and Docker to build a simple AI Agent, and expose it as a Web service.
Main Points
Using PhiData to build a simple AI Agent, which decides whether to turn on the heater or air conditioning based on temperature.
Using FastAPI to create a RESTful API, exposing the functionalities of the AI Agent.
Using Docker to containerize the entire application, enhancing the portability and scalability of the application.
This content was generated by AI summarization.

In this article, we will walk through the process of building an integrated AI Agent in Python using PhiData , FastAPI and Docker. The AI Agent will perform decision-making tasks, and we will use FastAPI to expose its functionalities as a Web service.Docker will be used to containerize the application for easier deployment and scaling.
The key components we will cover in this guide are:
- PhiData, for building and managing AI Agent workflows.
- FastAPI, used to create REST API for interacting with the Agent.
- Docker for containerizing the application to ensure portability and scalability.
1. Prerequisites
Before starting coding, ensure you have the following tools and libraries installed:
- Python (preferably 3.8 or higher)
- PhiData : for building AI agents.
- FastAPI : for creating RESTful API.
- Uvicorn : to serve FastAPI applications.
- Docker : to containerize applications.
Install Required Python Libraries:
pip install phidata fastapi uvicorn
Install Docker:
If you haven’t already, you can download and install Docker from here: https://www.docker.com/get-started
2. Building the AI Agent with PhiData
First, let’s define the AI Agent. We will build a simple Agent that decides whether to turn on the heater or air conditioning based on temperature.PhiData will be used to construct the logic and workflow of the agent.
Defining the Environment and Logic of the Agent
We will first simulate the environment in which the agent senses temperature, then decide whether to turn on the heater or air conditioning based on that temperature.
import phidata as pd
import random
import numpy as np
class TemperatureEnvironment: def __init__(self): self.temperature = 22 # Initial temperature in Celsius def get_state(self): """Return the current state of the environment (temperature).""" return np.array([self.temperature]) def update_state(self): """Simulate state changes (temperature fluctuations).""" self.temperature += random.uniform(-1, 1) # Random fluctuation def reset(self): """Reset environment to its initial state.""" self.temperature = 22
Defining the AI Agent
Now, we define the Agent that will use PhiData to make decisions. The Agent will decide whether to turn on the heater or air conditioning based on temperature.
class TemperatureControlAgent: def __init__(self, environment): self.environment = environment self.action_space = ['turn_on_heater', 'turn_on_ac', 'do_nothing'] # Define a task in PhiData that decides what action to take based on temperature self.task = pd.Task( name="Temperature Control", input_vars=["temperature"], output_vars=["action"], function=self.decide_action ) def decide_action(self, inputs): """Decide the best action based on the current state (temperature).""" temperature = inputs['temperature'] # Simple decision-making logic if temperature < 18: action = 'turn_on_heater' # Turn on the heater if it's too cold elif temperature > 25: action = 'turn_on_ac' # Turn on the AC if it's too hot else: action = 'do_nothing' # No action if the temperature is comfortable return {"action": action} def act(self): """Use the PhiData task to decide on an action.""" state = self.environment.get_state() inputs = {"temperature": state[0]} # Execute the task to decide the action result = self.task.execute(inputs) action = result["action"] return action
def train(self, steps=100): """Run the agent in the environment for a specified number of steps.""" for step in range(steps): # Make a decision action = self.act() print(f"Step {step}: Action: {action}") # Perform the action if action == 'turn_on_heater': print("Heater turned on.") elif action == 'turn_on_ac': print("AC turned on.") else: print("No action taken.") # Update the environment state self.environment.update_state()
Agent LogicSummary:
- Temperature Control :The Agent reads the current temperature and decides whether to turn on the heater, air conditioning, or do nothing.
- PhiData:The Agent uses PhiData through Task.task function to take the state of the environment as input and output the action the Agent should take.
3. Building the FastAPI Application
Now that we have defined the AI Agent, let’s use FastAPI to expose the Agent’s functionalities through RESTful API. This will allow external systems to interact with the Agent.
Defining the FastAPI Application
We will create a simple FastAPI application with an endpoint that allows users to query the agent’s actions based on the current temperature.
from fastapi import FastAPI
from pydantic import BaseModel
from typing import Optional
# Initialize FastAPI app
app = FastAPI()
# Define input model for FastAPI
class TemperatureRequest(BaseModel): temperature: float
# Initialize environment and agent
env = TemperatureEnvironment()
agent = TemperatureControlAgent(environment=env)
@app.post("/get_action/")
def get_action(request: TemperatureRequest): """ Endpoint to get the AI agent's decision based on the given temperature. """ env.temperature = request.temperature # Set the temperature in the environment action = agent.act() # Get the agent's action return {"action": action}
@app.get("/status/")
def get_status(): """ Endpoint to get the current state of the environment. """ state = env.get_state() return {"temperature": state[0]}
FastAPI Application Description:
- TemperatureRequest :A Pydantic model used to validate incoming data, ensuring temperature is passed as a float.
- /get_action/ :A POST endpoint that accepts temperature and returns the action the agent will take based on that temperature.
- /status/ :A GET endpoint to get the current temperature in the environment.
Running the FastAPI App
To run the FastAPI application locally, use Uvicorn:
uvicorn main:app --reload
Now, you can access the agent at http://127.0.0.1:8000 and test the Agent’s functionalities.The /get_action/ endpoint requires a JSON payload with temperature that will return the action the agent will perform.
Example Request:
curl -X 'POST' \
'http://127.0.0.1:8000/get_action/' \
-H 'Content-Type: application/json' \
-d '{ "temperature": 27}'
Example Response:
{ "action": "turn_on_ac"}
4. Containerizing the Application with Docker
To make our application portable and easy to deploy, we will use Docker to containerize it. This ensures that the application runs consistently across different environments.
Creating the Dockerfile
First, create a Dockerfile in your project directory:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install FastAPI, Uvicorn, and PhiData
RUN pip install --no-cache-dir fastapi uvicorn phidata
# Expose port 8000 for FastAPI app
EXPOSE 8000
# Run the FastAPI app using Uvicorn
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
Building the Docker Image
Run the following command to build and run the Docker container:
# Build the Docker image
docker build -t ai-agent-api .
# Run the Docker container
docker run -d -p 8000:8000 ai-agent-api
You can now access your containerized application by visiting http://localhost:8000 or by sending requests to the containerized application. The application is fully containerized and portable.
5. Summary
In this tutorial, we demonstrated how to create an AI Agent using the PhiData library in Python, expose it as a REST API using FastAPI, and containerize the entire application using Docker.
Key Takeaways:
- PhiData helps us model the decision logic of the AI Agent using simple tasks.
- FastAPI provides a straightforward way to expose the Agent’s functionalities through a RESTful API.
- Docker allows us to containerize the application, making it easy to deploy and scalable.
This architecture is highly scalable, allowing you to extend the capabilities of the agent, integrate it with other systems, or deploy it in a production environment with minimal changes.
Related Articles
Unveiling the Mysteries of Generative AI Agents: How to Build, Deploy, and Manage Agent Memory
A Comprehensive Introduction to AI Agents for Developers, Key Attributes, and Delegation Capabilities Among Agents
Understanding Agentic AI Architecture – A Comprehensive Guide for Beginners and Experts
Unveiling the Mysteries of AI Agents: 7 Common FAQs: Definitions, Types, Application Ecosystem
2025 Trends in Enterprise Data and AI: AI Agents, Intelligent Data Platforms, Data and Operations Trends
Human Intelligence Exchange
Bringing Future Intelligence Closer
Facilitating Communication Platform Between Humanity and Future Intelligence
All Networks | Human Intelligence Exchange
Join the Communication Group ·
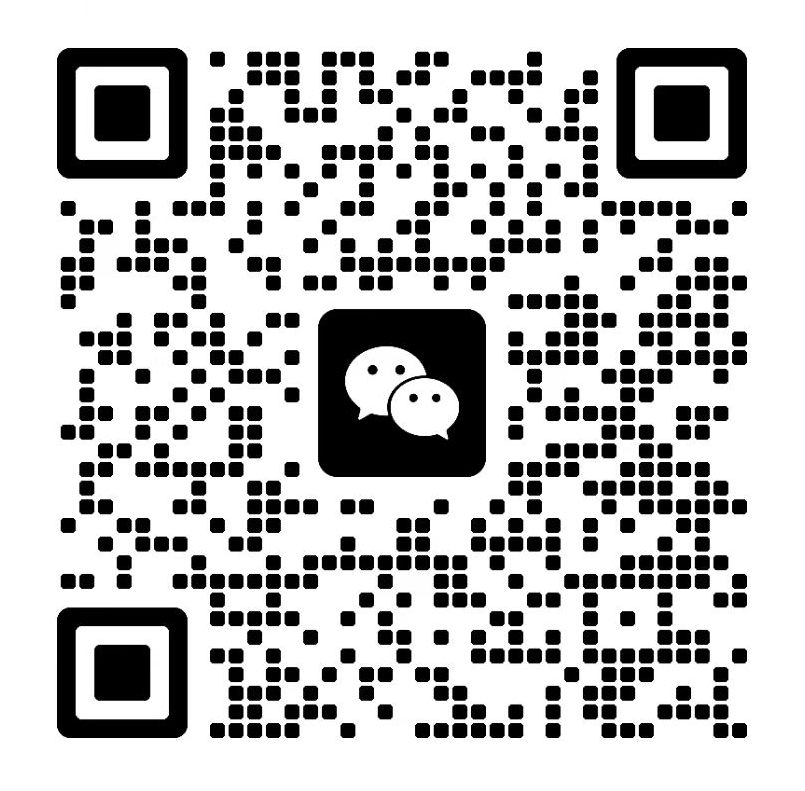
![]() |
AIGC Communication Group · |
![]() |
Office Application Communication Group |
![]() |
AI Programmer Communication Group |