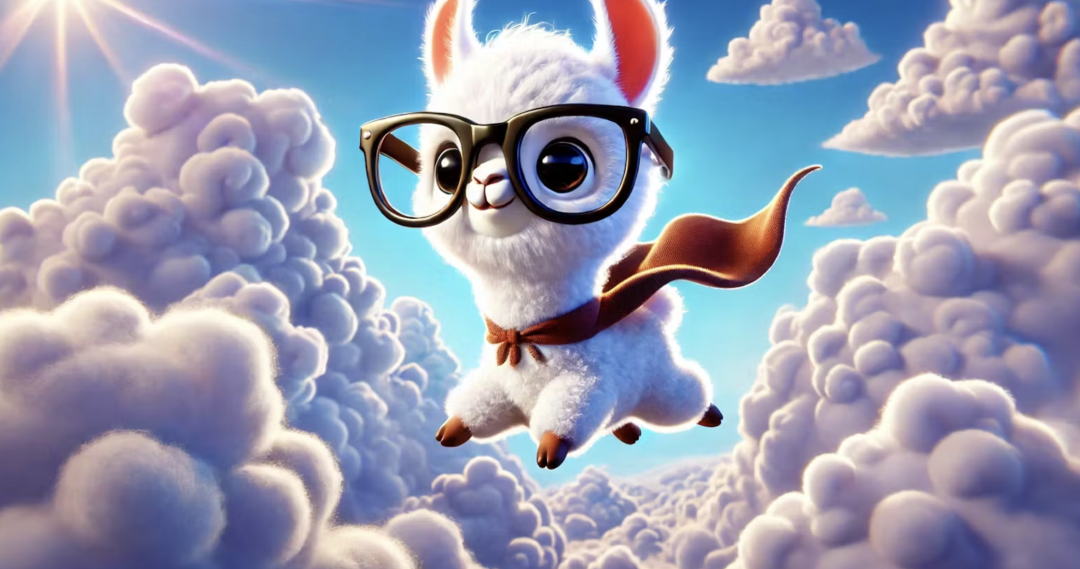
The MCP is an open protocol that standardizes how applications provide context to large language models (LLMs). It also provides a standardized way to connect AI models to various data sources and tools.
This protocol was defined by Anthropic, and one of its first implementations is Claude Desktop. This application allows Anthropic’s Claude AI model to interact with your computer using the MCP protocol.
General Architecture
The Model Context Protocol (MCP) uses a client-server architecture where the host application can connect to multiple servers:
MCP Host: These are generative AI applications that use LLMs and wish to access external resources via MCP. Claude Desktop is an example of a host application.
MCP Client: A protocol client that maintains a 1:1 connection with the server (the client is used by the MCP host application).
MCP Server: A program that exposes specific functionalities using local or remote data sources through the MCP protocol.
The MCP protocol provides two main transport models: STDIO (standard input/output) and SSE (server-sent events). Both use JSON-RPC 2.0 as the message format for data transmission.
The first model, STDIO, communicates via standard input/output streams. It is suitable for local integration. The second model, SSE, uses HTTP requests for communication, where SSE is used for server-to-client communication and POST requests are used for client-to-server communication. It is more suitable for remote integration.
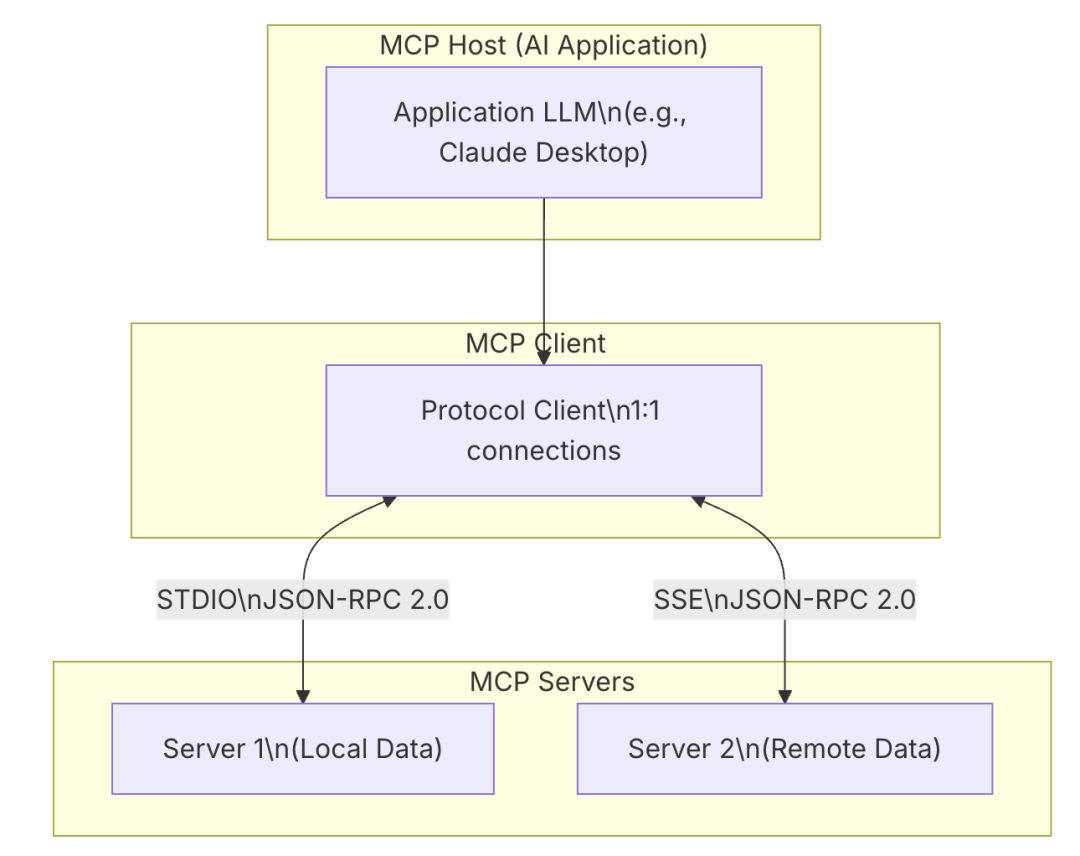
tools: [ { name: "add_numbers", description: "Add two numbers together", inputSchema: { type: "object", properties: { a: { type: "number" }, b: { type: "number" } }, required: ["a", "b"] } }, { name: "subtract_numbers", description: "Subtract two numbers together", inputSchema: { type: "object", properties: { a: { type: "number" }, b: { type: "number" } }, required: ["a", "b"] } }]
"tools": [ { "function": { "description": "Add two numbers", "name": "add_numbers", "parameters": { "properties": { "number1": { "description": "The first number", "type": "number" }, "number2": { "description": "The second number", "type": "number" } }, "required": [ "number1", "number2" ], "type": "object" } }, "type": "function" }, { "function": { "description": "Subtract two numbers", "name": "subtract_numbers", "parameters": { "properties": { "number1": { "description": "The first number", "type": "number" }, "number2": { "description": "The second number", "type": "number" } }, "required": [ "number1", "number2" ], "type": "object" } }, "type": "function" }, ]}
Using the tools list, the host application can generate a prompt for the model. For example, tools list + “Add 12 and 28”.
If the large language model (LLM) supports tools, it can understand this prompt, extract the numbers, recognize that this is an addition operation, and respond with this type of JSON result:
"tool_calls": [ { "function": { "name": "add_numbers", "arguments": { "number1": 12, "number2": 28 } } }]
{ "name":"add_numbers", "arguments": { "number1":28, "number2":14 }}
{ "result": 42}
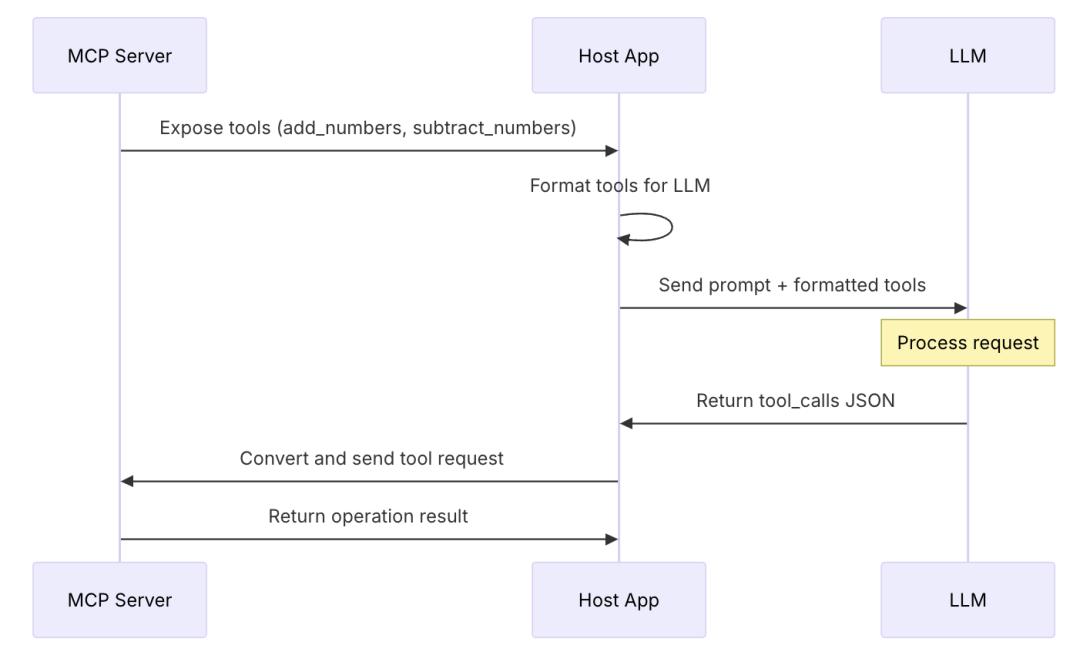
The main advantages of MCP
-
Standardization: A unified way to connect LLMs to different data sources.
-
Flexibility/Scalability:
1. The ability to switch LLM providers.
2. The ability to change, add, or modify MCP servers.
-
Security: Protecting data within your infrastructure.
-
Reusability: MCP servers can be used across different projects.
Using the MCP server available on Docker Hub with Claude Desktop provides a ready-made MCP server that can be found at https://hub.docker.com/u/mcp. For example, the SQLite MCP server can be found at https://hub.docker.com/r/mcp/sqlite.
To use this MCP server from Docker Hub with Claude Desktop, you need to add a configuration in the claude_desktop_config.json file to tell Claude Desktop how to start the MCP server. Here is an example configuration for the SQLite MCP server:
{ "mcpServers": { "sqlite": { "command": "docker", "args": [ "run", "--rm", "-i", "-v", "mcp-test:/mcp", "mcp/sqlite", "--db-path", "/mcp/test.db" ] } }}
You can add multiple MCP servers to this configuration. For more information on Claude Desktop configuration, please refer to the official documentation: https://modelcontextprotocol.io/quickstart/user
This will allow you to see the available tools in Claude Desktop:
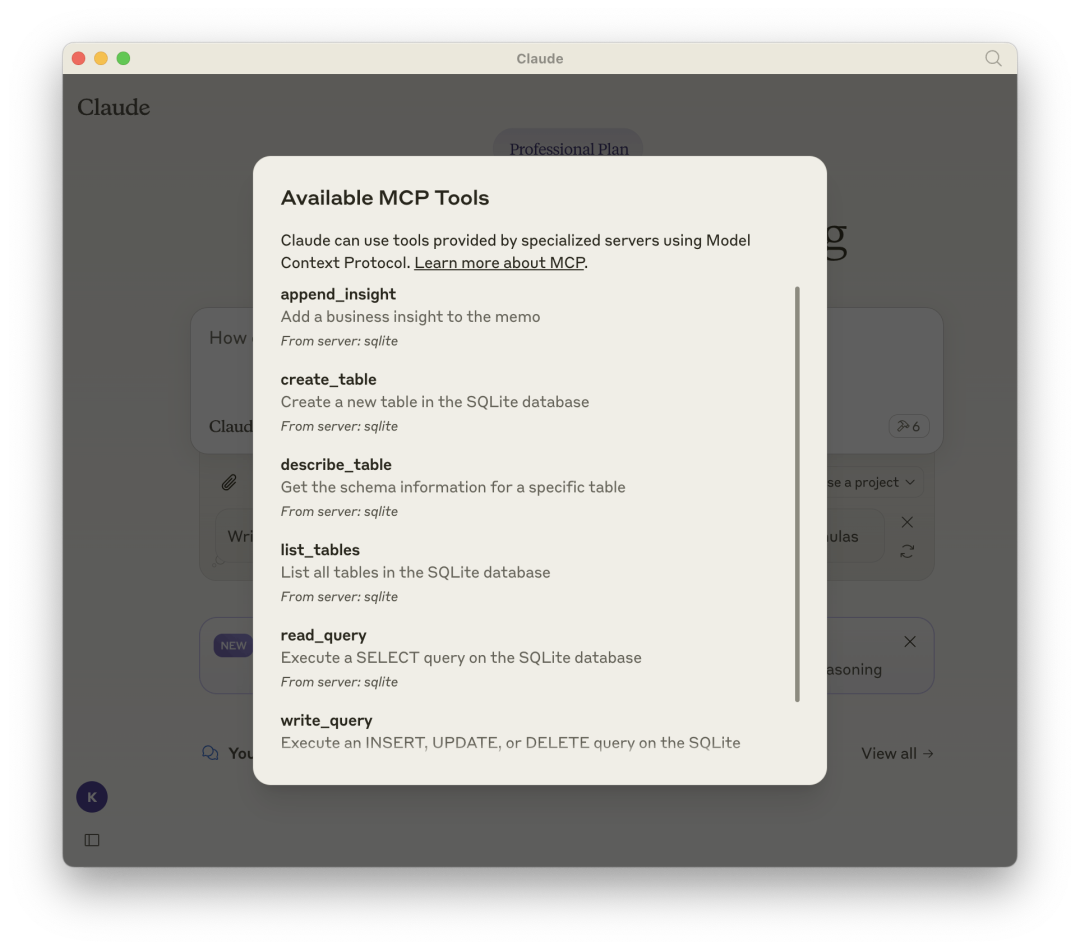
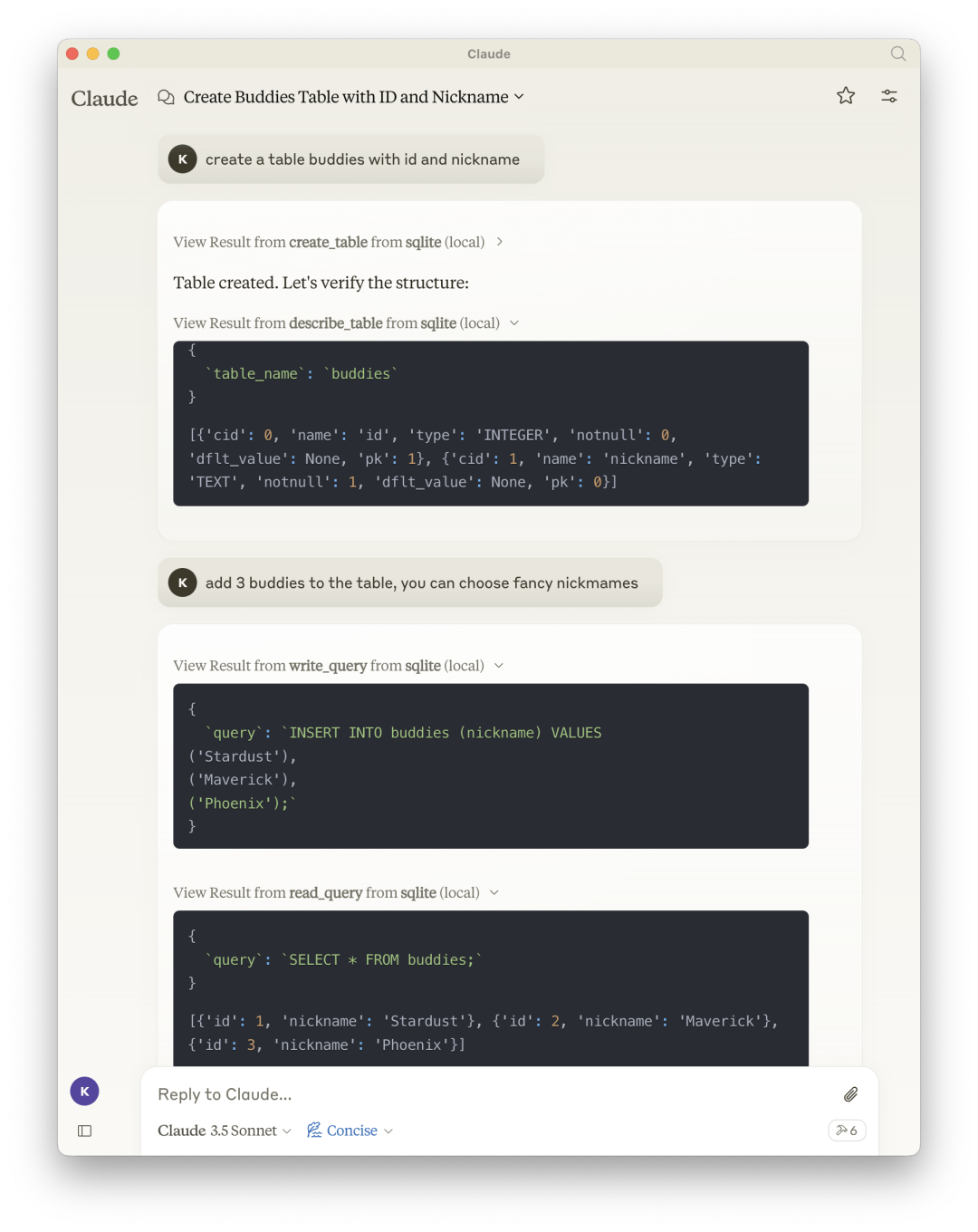
In this example, I requested Claude Desktop to create a table named buddies in the SQLite database and add 3 records to it.
✋ Note that the same operation can also be done using a locally hosted LLM provided by Ollama.
Using the SQLite MCP server with Ollama and local LLM Since I particularly like the Go language, I searched for an MCP CLI written in Go and found mcphost from Mark3Labs ( https://github.com/mark3labs/mcphost ). You can install it with the following command:
go install github.com/mark3labs/mcphost@latest
Next, create a configuration file <span>mcp.json</span>
to tell <span>mcphost</span>
how to start the SQLite MCP server:
{ "mcpServers": { "sqlite": { "command": "docker", "args": [ "run", "--rm", "-i", "-v", "mcp-test:/mcp", "mcp/sqlite", "--db-path", "/mcp/test.db" ] } }}
You can now start mcphost with the following command
mcphost --config ./mcp.json --model ollama:qwen2.5:3b
Of course, you need to use the command <span>ollama pull qwen2.5:3b</span>
to download the qwen2.5:3b model.
Now, you can interact with the SQLite MCP server.
The command line interface will display the available MCP servers, and you can enter your prompts:
You can use the command <span>/tools</span>
to request a list of available tools:
Then interact with the available tools. Here, I requested to create a table named users in the SQLite database and add three records to it:
And just like that, the users table has been successfully created, and three records have been added. I can now request to see the user list:
The result is as follows:
That’s it! Now you know how to use the Model Context Protocol (MCP) with Claude Desktop and Ollama. As you can see, implementing an MCP server using Docker is very simple and quick. You can now explore the various possibilities of the MCP servers available at https://hub.docker.com/u/mcp, connecting your large language models (LLMs) to external information sources.
You can find the source code for the official MCP server here: https://github.com/modelcontextprotocol/servers
In an upcoming blog post, I will explain how to create your own MCP server and how to develop host applications to interact with this MCP server.