Are you confused about the differences between params and query, and their usage scenarios? Don’t worry, I will explain the usage techniques of these two parameter passing methods in detail! π
1. Basic Knowledge of Vue Router
Before diving into params and query, let’s first understand the basic concept of Vue Router. Vue Router is the official router manager for Vue.js, allowing us to achieve different view switches in a single-page application. Through routing, we can map different URLs to different components, thus achieving dynamic page effects.
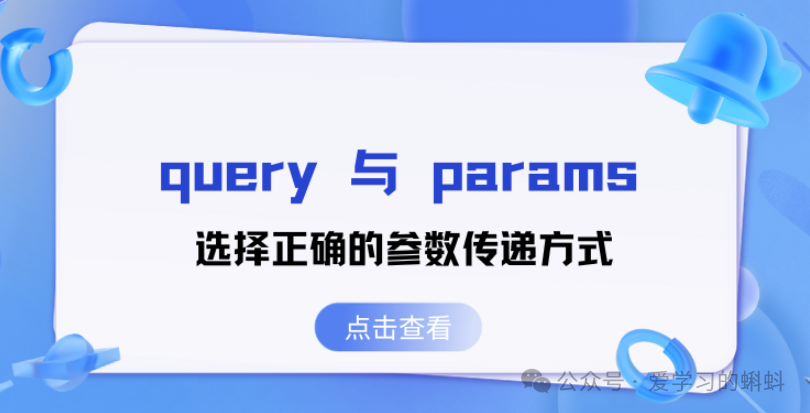
1.1 Basic Configuration of Routing
Before using Vue Router, we need to perform basic configuration. Here is a simple routing configuration example:
import Vue from 'vue';
import Router from 'vue-router';
import Home from './components/Home.vue';
import User from './components/User.vue';
Vue.use(Router);
const router = new Router({
routes: [
{ path: '/', component: Home },
{ path: '/user/:id', component: User }
]
});
export default router;
In this configuration, we defined two routes: one is the homepage (<span>/</span>
), and the other is the user page (<span>/user/:id</span>
), where <span>:id</span>
is a dynamic parameter.
2. Differences Between Params and Query
In Vue Router, both params and query are ways to pass parameters, but their usage scenarios and characteristics differ.
2.1 Params
Params are parameters passed through the route path, typically used for dynamic data that needs to be clearly represented in the URL. The characteristics of params include:
-
Path Parameters: params are part of the URL, usually starting with <span>:</span>
, for example,<span>/user/:id</span>
. -
Strong Typing: The values of params are parsed when the route is matched, making them suitable for scenarios that require strong typing. -
No Duplicate Parameters: Params cannot be duplicated within the same route.
Usage Example
Suppose we have a user detail page, we can pass the user ID through params:
// Route configuration
{ path: '/user/:id', component: User }
// Navigate to user page
this.$router.push({ path: `/user/${userId}` });
In the user component, we can access the passed parameters through <span>this.$route.params</span>
:
export default {
mounted() {
const userId = this.$route.params.id;
console.log(`User ID: ${userId}`);
}
}
2.2 Query
Query are parameters passed through the URL query string, typically used for optional parameters or scenarios with multiple parameters. The characteristics of query include:
-
Query String: query is part of the URL, formatted as <span>?key=value</span>
, and can include multiple parameters, for example,<span>/user?id=1&name=John</span>
. -
Flexibility: query parameters can be optional, making them suitable for filtering, sorting, and other scenarios. -
Supports Duplicate Parameters: Query can repeat within the same route.
Usage Example
If we want to pass multiple parameters, such as user ID and user name, we can use query:
// Route configuration
{ path: '/user', component: User }
// Navigate to user page
this.$router.push({ path: '/user', query: { id: userId, name: userName } });
In the user component, we can access the passed parameters through <span>this.$route.query</span>
:
export default {
mounted() {
const userId = this.$route.query.id;
const userName = this.$route.query.name;
console.log(`User ID: ${userId}, User Name: ${userName}`);
}
}
3. Usage Scenarios of Params and Query
After understanding the basic concepts and usage methods of params and query, let’s look at their respective suitable usage scenarios.
3.1 Scenarios for Using Params
-
Resource Identification: When you need to explicitly identify a resource through the URL, such as user ID or article ID, params is a good choice. -
Data Advantages: Since params is part of the URL, search engines can more easily crawl this information.
3.2 Scenarios for Using Query
-
Optional Parameters: When you need to pass some optional parameters, such as filtering conditions or sorting methods, query is more flexible. -
Multiple Parameters: If you need to pass multiple parameters, query can facilitate this.
4. Comparison of Advantages and Disadvantages of Params and Query
|
|
|
---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
5. Conclusion
If you have any other questions about Vue Router, feel free to leave a message in the comments section, and we can discuss it together! π¬
Related Articles
γOpenAIγ(1) A Comprehensive Guide to Obtaining OpenAI API Key: From Beginner to Expert, and Detailed Tutorials!!
γVSCodeγ(2) The Powerful AI-GPT Programming Tool in VSCode, Fully Revealing CodeMoss & ChatGPT Chinese Version
γCodeMossγ(3) Integrating 13 AI Large Models (GPT4, o1, etc.), Supporting Open API Calls, Custom Assistants, File Uploads, and More Powerful Features to Boost Your Work Efficiency! >>> – CodeMoss & ChatGPT-AI Chinese Version
π Experience GPT-AI Large Models for Free – ChatMoss & ChatGPT Chinese Version π π Click the link to register: https://pc.aihao123.cn/index.html#/page/login?invite=1141439&fromChannel=gzh_CodeMoss
π Register to enjoy 3 days of free premium model experience! Just complete the beginner tasks to gain another 3 days of usage time! β¨
π Learn more about the usage introduction of CodeMoss: π Click here to view: https://blog.csdn.net/zhouzongxin94/article/details/143489698
Come and experience unprecedented intelligent conversations! π€π¬