Source: Authorized reproduction from Machine Learning Algorithms and Python Practice
Author: Lao Zhang is Busy
Discovered an amazing Python library that makes creating large model applications incredibly simple.
8 lines of code is enough (with 2 optional lines).
import gradio as gr
import ai_gradio
gr.load(
name='qwen:qwen1.5-14b-chat',
src=ai_gradio.registry,
title='AI Chat',
description='Chat with an AI model'
).launch()
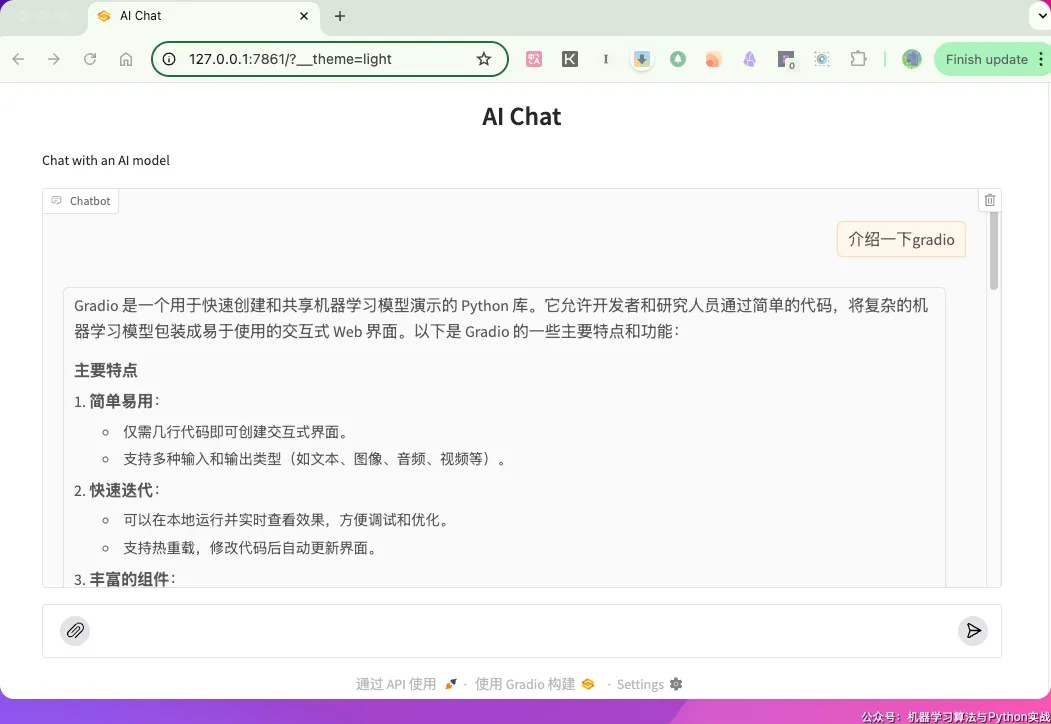
It also has voice chat, video chat, camera mode, and browser automation features, making it powerful and extremely easy to use.
Project address: https://github.com/AK391/ai-gradio
❝
ai-gradio is a Python package that helps developers easily create machine learning applications powered by various AI providers. It is built on Gradio and provides a unified interface for multiple AI models and services.
Key Highlights
-
Feature-Rich: ai-gradio supports multiple providers, integrating over 15 AI providers including OpenAI, Google Gemini, Anthropic, and more. It features text chat, voice chat, video chat, code generation, multi-modal support, agent teams, and browser automation. -
Easy Installation: You can install the core package of ai-gradio and specific provider support packages via pip. There are various installation options, such as installing support for a single provider or all providers. -
Diverse Usage Examples: It provides methods for API key configuration, quick start examples, and advanced feature examples, including creating different types of chat interfaces, coding assistants, multi-provider interfaces, CrewAI teams, browser automation, and Swarms integration.
Core Language Models
Provider | Models |
---|---|
OpenAI | gpt-4-turbo, gpt-4, gpt-3.5-turbo |
Anthropic | claude-3-opus, claude-3-sonnet, claude-3-haiku |
Gemini | gemini-pro, gemini-pro-vision, gemini-2.0-flash-exp |
Groq | llama-3.2-70b-chat, mixtral-8x7b-chat |
Specialized Models
Provider | Type | Models |
---|---|---|
LumaAI | Generation | dream-machine, photon-1 |
DeepSeek | Multi-purpose | deepseek-chat, deepseek-coder, deepseek-vision |
CrewAI | Agent Teams | Support Team, Article Team |
Qwen | Language | qwen-turbo, qwen-plus, qwen-max |
Browser | Automation | browser-use-agent |
Installation and Usage
Installation is straightforward, pip install ai-gradio
is a must, and you also need to install additional large model support packages. For example, I only installed pip install 'ai-gradio[deepseek]'
and pip install 'ai-gradio[qwen]'
. If you want to save some trouble, you can also install everything at once with pip install 'ai-gradio[all]'
.
# Install core package
pip install ai-gradio
# Install with specific provider support
pip install 'ai-gradio[openai]' # OpenAI support
pip install 'ai-gradio[gemini]' # Google Gemini support
pip install 'ai-gradio[anthropic]' # Anthropic Claude support
pip install 'ai-gradio[groq]' # Groq support
pip install 'ai-gradio[crewai]' # CrewAI support
pip install 'ai-gradio[lumaai]' # LumaAI support
pip install 'ai-gradio[xai]' # XAI/Grok support
pip install 'ai-gradio[cohere]' # Cohere support
pip install 'ai-gradio[sambanova]' # SambaNova support
pip install 'ai-gradio[hyperbolic]' # Hyperbolic support
pip install 'ai-gradio[deepseek]' # DeepSeek support
pip install 'ai-gradio[smolagents]' # SmolagentsAI support
pip install 'ai-gradio[fireworks]' # Fireworks support
pip install 'ai-gradio[together]' # Together support
pip install 'ai-gradio[qwen]' # Qwen support
pip install 'ai-gradio[browser]' # Browser support
# Install all providers
pip install 'ai-gradio[all]'
Just to give an example with Tongyi Qianwen.
Model list: https://bailian.console.aliyun.com/?spm=a2c4g.11186623.0.0.6f94b0a8AKJSUG#/model-market
API acquisition: https://bailian.console.aliyun.com/#/home
Select API-KEY in the upper right corner of the console, then create one for API calls to the large model.
Copy it for later use.
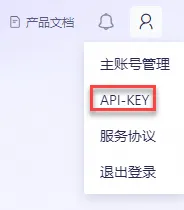
As a side note, below is an example of calling the Tongyi Qianwen API using Python with Qwen, which is very convenient.
import os
from openai import OpenAI
client = OpenAI(
# If you haven't configured the environment variable, replace the following line with: api_key="sk-xxx",
api_key=os.getenv("DASHSCOPE_API_KEY"), # How to get API Key: https://help.aliyun.com/zh/model-studio/developer-reference/get-api-key
base_url="https://dashscope.aliyuncs.com/compatible-mode/v1",
)
completion = client.chat.completions.create(
model="qwen-plus", # Model list: https://help.aliyun.com/zh/model-studio/getting-started/models
messages=[
{'role': 'system', 'content': 'You are a helpful assistant.'},
{'role': 'user', 'content': 'Who are you?'}
]
)
print(completion.choices[0].message.content)
For the ai-gradio example, you need to set the API key in advance.
import os
os.environ["DASHSCOPE_API_KEY"] = "sk-上面复制好的"
In fact, ai-gradio also has a window mode, as mentioned above, just prepare the API in advance, and you can switch between text, image, and code modes on one page.
import gradio as gr
import ai_gradio
with gr.Blocks() as demo:
with gr.Tab("Text"):
gr.load('openai:gpt-4-turbo', src=ai_gradio.registry)
with gr.Tab("Vision"):
gr.load('deepseek:deepseek-vision', src=ai_gradio.registry)
with gr.Tab("Code"):
gr.load('deepseek:deepseek-coder', src=ai_gradio.registry)
demo.launch()
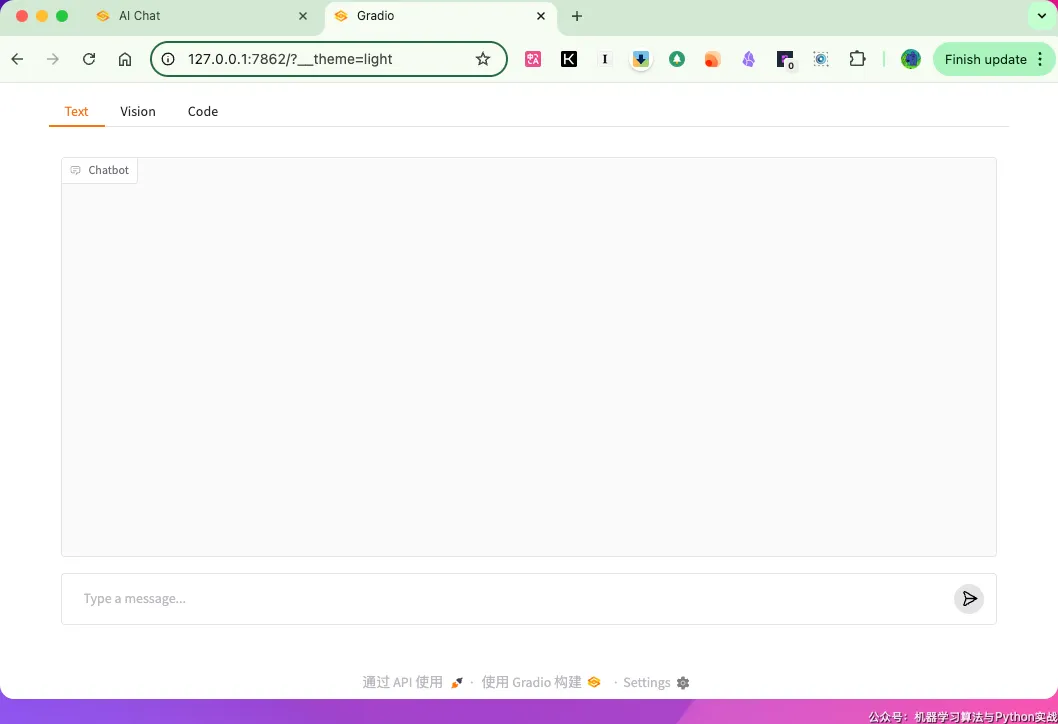
Voice input and camera mode I haven’t explored in depth.
Just tried it briefly.
gr.load(
name='openai:gpt-4-turbo',
src=ai_gradio.registry,
enable_voice=True,
title='AI Voice Assistant'
).launch()
Error message:
HTTP Error Your request was:
POST /Accounts/None/Tokens.json
Twilio returned the following information:
Unable to create record: Authentication Error - No credentials provided
More information may be available here:
https://www.twilio.com/docs/errors/20003
Found the reason, both camera and voice rely on browser-use.
Project address:
https://github.com/browser-use/browser-use
From the official example, it seems quite powerful; I will try it again when I have the chance.
import asyncio
import os
from langchain_ollama import ChatOllama
from browser_use import Agent
async def run_search():
agent = Agent(
task=(
'1. Go to https://www.reddit.com/r/LocalLLaMA'
"2. Search for 'browser use' in the search bar"
'3. Click search'
'4. Call done'
),
llm=ChatOllama(
# model='qwen2.5:32b-instruct-q4_K_M',
# model='qwen2.5:14b',
model='qwen2.5:latest',
num_ctx=128000,
),
max_actions_per_step=1,
tool_call_in_content=False,
)
await agent.run()
if __name__ == '__main__':
asyncio.run(run_search())