Today, I want to share an interesting development experience where, using Cursor, this magical AI coding assistant, we helped a programming novice rapidly develop a simple social application in just three hours.
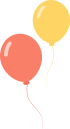
1.
Act One: Getting to Know Cursor
“Your Majesty, I want to create a simple social application, but I only know a little Python…” the concubine whispered.
“Don’t worry, with me and Cursor here! Let’s first install this magical coding assistant.”
“Wow, is it an editor similar to VS Code?”
“Exactly, and it’s even more powerful than ordinary editors. Now, let’s create a new project.”
# Create project directory structure
project/
├── app.py
├── templates/
│ ├── index.html
│ └── profile.html
└── static/
└── style.css
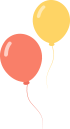
2.
Act Two: Rapidly Building the Framework
“Your Majesty, how do I write so many files?” the concubine looked at the empty files, bewildered.
“Watch me! Press Ctrl + L to summon the Cursor assistant. First, we tell it we want a simple social application.”
I typed in app.py:
# Create a simple Flask social application with user registration, login, and posting features
Amazing things happened, Cursor immediately provided the complete framework code:
from flask import Flask, render_template, request, redirect, session
from flask_sqlalchemy import SQLAlchemy
from werkzeug.security import generate_password_hash, check_password_hash
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///social.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
password = db.Column(db.String(120), nullable=False)
posts = db.relationship('Post', backref='author', lazy=True)
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
content = db.Column(db.String(500), nullable=False)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
“This is incredible! We now have the database model!” the concubine exclaimed.
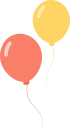
3.
Act Three: Enhancing Functionality
“Next, we need to add routes and view functions.” I continued using Cursor’s smart completion feature.
@app.route('/')
def index():
posts = Post.query.all()
return render_template('index.html', posts=posts)
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = generate_password_hash(request.form['password'])
user = User(username=username, password=password)
db.session.add(user)
db.session.commit()
return redirect('/login')
return render_template('register.html')
“Your Majesty, what about the frontend page?”
“Cursor can also help us generate templates, look at this:”
<!-- templates/index.html -->
<!DOCTYPE html>
<html>
<head>
</head>
<body>
{% if session.get('user_id') %}
<button>Post</button>
{% endif %}
{% for post in posts %}
<div>
{{ post.author.username }} says:
{{ post.content }}
</div>
{% endfor %}
</body>
</html>
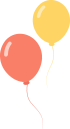
4.
Act Four: Refining and Perfecting
“Let’s add some styles!” I said cheerfully.
/* static/style.css */
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
.post {
border: 1px solid #ddd;
margin: 10px 0;
padding: 15px;
border-radius: 5px;
}
textarea {
width: 100%;
height: 100px;
margin: 10px 0;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
“Wow, now it has styles!” the concubine said happily.
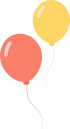
5.
The Final Touch
“Let’s use Cursor’s code optimization feature to check if there are any improvements to be made.”
With that, I pressed Ctrl + Shift + P and selected “Improve Code”, and Cursor immediately provided optimization suggestions:
-
Add error handling -
Increase user input validation -
Optimize database query performance
Three hours passed in the blink of an eye, and a simple but fully functional social application was born!
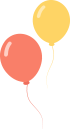
6.
Conclusion
Through this development, we experienced the powerful features of Cursor:
-
Intelligent code generation -
Code completion hints -
Automatic error detection -
Code optimization suggestions
For programming novices, Cursor is like a caring teacher, not only helping you write code but also teaching you better programming practices. Remember, no matter how powerful Cursor is, it’s just a tool; the key is to practice more, think more, and turn this knowledge into your own ability.
“Thank you for your guidance, Your Majesty!” the concubine said happily, “With Cursor, programming has really become so much fun!”
“Go try developing your own project, I believe you can do even better!”