Hello everyone, today we are going to learn how to use MetaGPT to implement a practical case of multi-agent collaboration – the Werewolf game. Werewolf is a classic social deduction game, and through MetaGPT’s multi-agent framework, we can simulate the behaviors of characters such as werewolves, villagers, guards, seers, and witches, and let them interact in the game. Next, we will guide you step by step through the game rules, code implementation, and problem fixes for this interesting practical project.
Introduction to Game Rules
The Werewolf game is a social deduction game involving multiple participants, with roles divided into three main categories: werewolves, villagers, and special roles. The basic rules are as follows:
-
Role Assignment: Before the game starts, each player is randomly assigned a role, including werewolves, ordinary villagers, and special ability villagers (such as seers, witches, hunters, etc.). -
Game Process: The game consists of two phases: night and day. At night, werewolves open their eyes and kill one player; during the day, all players discuss and vote to execute one player. This process continues until a victory condition is met. -
Victory Conditions:
-
Werewolf Victory: The werewolf camp wins when the number of werewolves equals the number of villagers. -
Villager Victory: The villager camp wins when all werewolves are identified and executed.
MetaGPT Multi-Agent Framework
In MetaGPT, the core focus of the Werewolf game is on three parts:
-
Role: The roles of the agents, such as villagers, werewolves, guards, seers, witches, etc. -
Action: Actions corresponding to the roles, such as the werewolf’s “Hunt”, the guard’s “Protect”, and the seer’s “Verify”. -
Interaction Environment: The environment connects messages between roles, enabling information exchange among agents.
Role Definition
All roles inherit from the <span>BasePlayer</span>
class, which encapsulates the basic behaviors and attributes of the roles. For example:
-
Villager: Has the <span>Speak</span>
behavior. -
Werewolf: Besides <span>Speak</span>
, has special skills<span>Hunt</span>
(to kill) and<span>Impersonate</span>
(to disguise). -
Guard: Has the <span>Protect</span>
skill. -
Seer: Has the <span>Verify</span>
skill. -
Witch: Has <span>Save</span>
and<span>Poison</span>
skills.
Action Definition
Each role has specific actions. For example, werewolves perform the <span>Hunt</span>
action at night and disguise themselves as good people during the day. Guards perform the <span>Protect</span>
action at night to protect other players.
Environment Definition
The environment (<span>WerewolfEnv</span>
) is used to pass messages between roles and control the rounds and states of the game. The environment is also responsible for updating the game and the states of each role.
Code Implementation
We create a <span>werewolf.py</span>
file, with the following code:
import asyncio
import fire
from metagpt.ext.werewolf.roles import Guard, Moderator, Seer, Villager, Werewolf, Witch
from metagpt.ext.werewolf.roles.human_player import prepare_human_player
from metagpt.ext.werewolf.werewolf_game import WerewolfGame
from metagpt.logs import logger
async def start_game(
investment: float = 3.0,
n_round: int = 5, # It is recommended to set a smaller value
shuffle: bool = True,
add_human: bool = False,
use_reflection: bool = True,
use_experience: bool = False,
use_memory_selection: bool = False,
new_experience_version: str = "",
):
game = WerewolfGame()
game_setup, players = game.env.init_game_setup(
role_uniq_objs=[Villager, Werewolf, Guard, Seer, Witch],
num_werewolf=2,
num_villager=2,
shuffle=shuffle,
add_human=add_human,
use_reflection=use_reflection,
use_experience=use_experience,
use_memory_selection=use_memory_selection,
new_experience_version=new_experience_version,
prepare_human_player=prepare_human_player,
)
logger.info(f"{game_setup}")
players = [Moderator()] + players # Moderator joins the game
game.hire(players)
game.invest(investment)
game.run_project(game_setup) # Moderator broadcasts the game status
await game.run(n_round=n_round)
def main(
investment: float = 20.0,
n_round: int = 12, # It is recommended to set a smaller value
shuffle: bool = True,
add_human: bool = False,
use_reflection: bool = True,
use_experience: bool = False,
use_memory_selection: bool = False,
new_experience_version: str = "",
):
asyncio.run(
start_game(
investment,
n_round,
shuffle,
add_human,
use_reflection,
use_experience,
use_memory_selection,
new_experience_version,
)
)
if __name__ == "__main__":
fire.Fire(main)
Run the command <span>python werewolf.py</span>
in the terminal, and it will print the game-related messages.
Common Issues and Fixes
1. Runtime Error: <span>No module named 'metagpt.ext'</span>
Cause: The <span>metagpt</span>
installed via <span>pip</span>
does not package the <span>ext</span>
directory.
Solution:
-
Get the <span>metagpt/ext</span>
directory from GitHub: MetaGPT GitHub. -
Copy the <span>werewolf</span>
directory to the<span>pip</span>
installed<span>metagpt</span>
directory. -
Install the corresponding <span>llama-index</span>
dependency: Issue #1653.
2. Runtime Error: <span>IndexError: list index out of range</span>
Cause: The list returned by <span>self.rc.memory.get()</span>
is empty, causing an error when accessing the <span>[-1]</span>
index.
Solution: Modify the code as follows:
memory = self.rc.memory.get()
if not memory:
return False
3. Runtime Error: <span>Cannot import Url from pydantic.networks</span>
Cause: Incompatible version of <span>pydantic</span>
.
Solution: Downgrade <span>pydantic</span>
to <span>2.9.2</span>
:
pip install pydantic==2.9.2
Running Results:
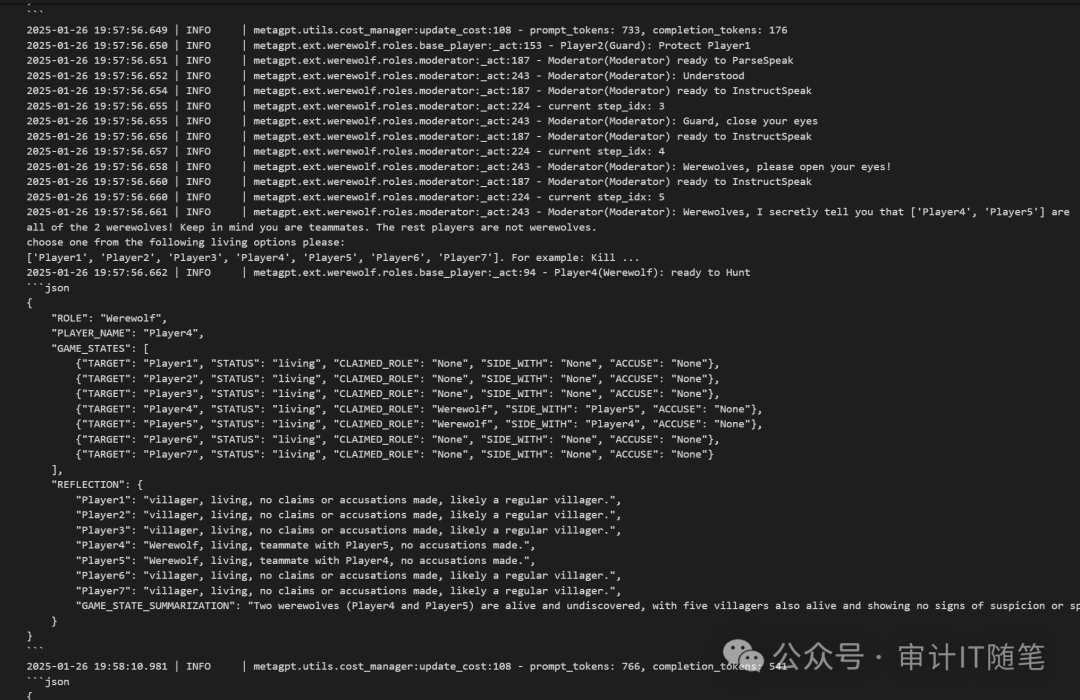
Token Consumption
Conclusion
Through MetaGPT’s multi-agent framework, we can easily simulate the Werewolf game. This article detailed how to complete this project, from game rules and code implementation to problem fixes. If you are interested in multi-agent collaboration, why not try this case and experience the interaction and competition among agents!