I feel quite embarrassed discussing this topic. I have switched personal knowledge management software several times, from WizNote, Evernote to Notion, Craft. The ultimate reason is not that the software is not good, but mainly because my habits and methods were incorrect. Since last year, I have mainly been using two software: Obsidian and Craft, while continuing to use Typora for Markdown writing. I chose Craft mainly for family use, as it syncs easily with the iPad at home, allowing everyone, including kids, to participate in making travel plans. On the other hand, Obsidian is primarily for my personal knowledge management, and it works quite smoothly with plugins.
1.
The Plugin System Worth Exploring
The main issue with Notion is that it cannot work offline (limited offline support is only partial caching), while Obsidian, on the other hand, is completely localized for storage. Even syncing requires plugins or a paid official service.
How powerful is the Obsidian plugin system? Let’s put it this way: if you are familiar with JavaScript/TypeScript, you can create an IDE inside Obsidian. Plugins are divided into official plugins and community plugins, with the community plugins being numerous and varying in quality, requiring users to choose suitable plugins themselves.
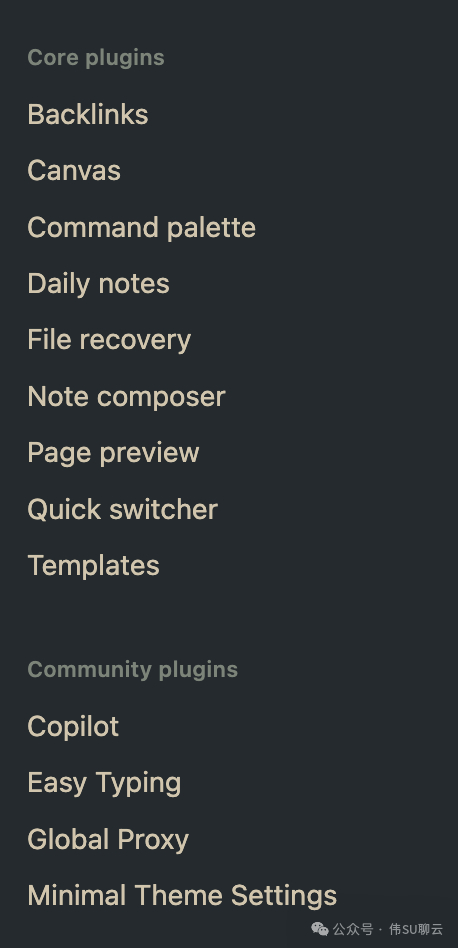
2.
Obsidian-Copilot
There are several community plugins for AI writing in Obsidian. After trying a few, I chose obsidian-copilot for several reasons:
1. It supports multiple models. The original project supports model providers like OpenAI, Anthropic, Azure, and also allows custom model settings (needs to be compatible with OpenAI API, such as Ollama).
2. It supports embedding functionality for local Markdown files.
Based on these reasons, I chose to do secondary development on it to add support for Amazon Bedrock. Let’s first look at the final effect:
Copilot configuration interface allows selecting from four Amazon Bedrock models:
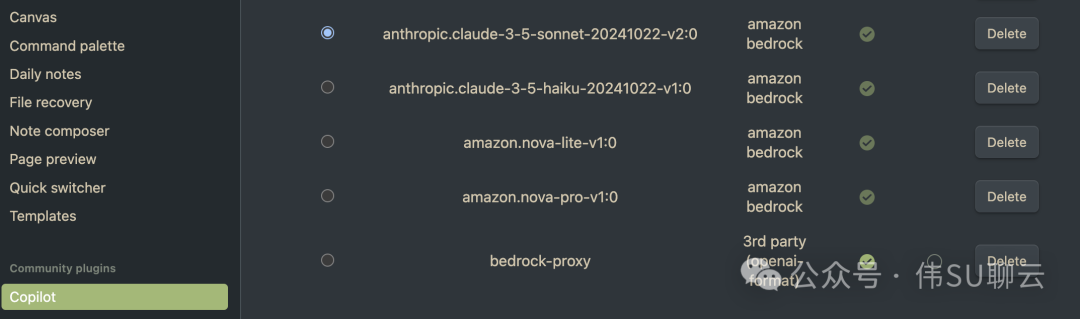
We can let it summarize an article:
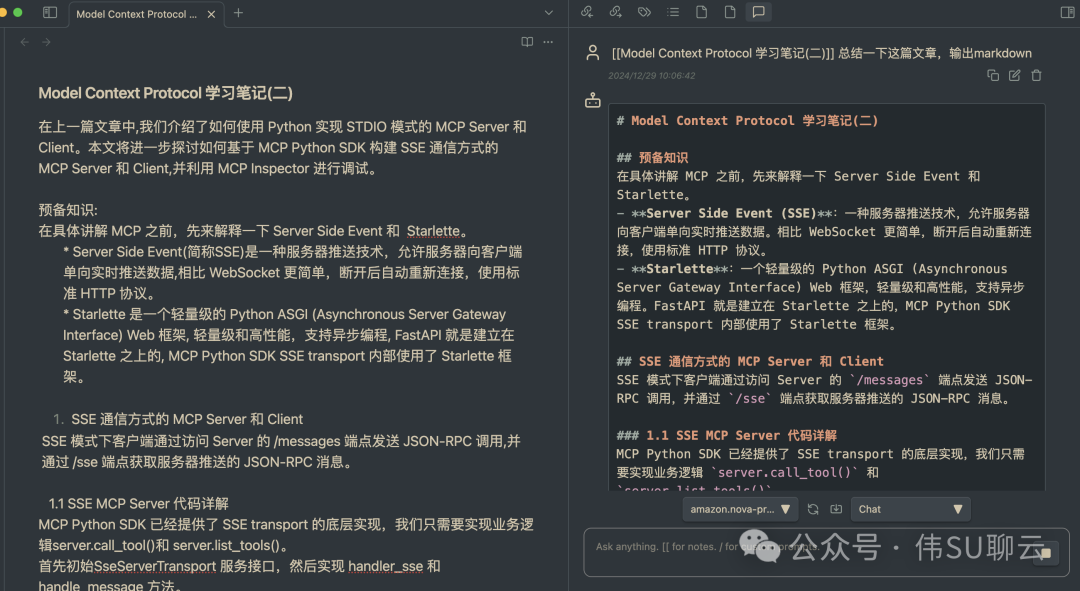
It can also output in Markdown format, and with the Mindmap plugin, directly generate mind maps.
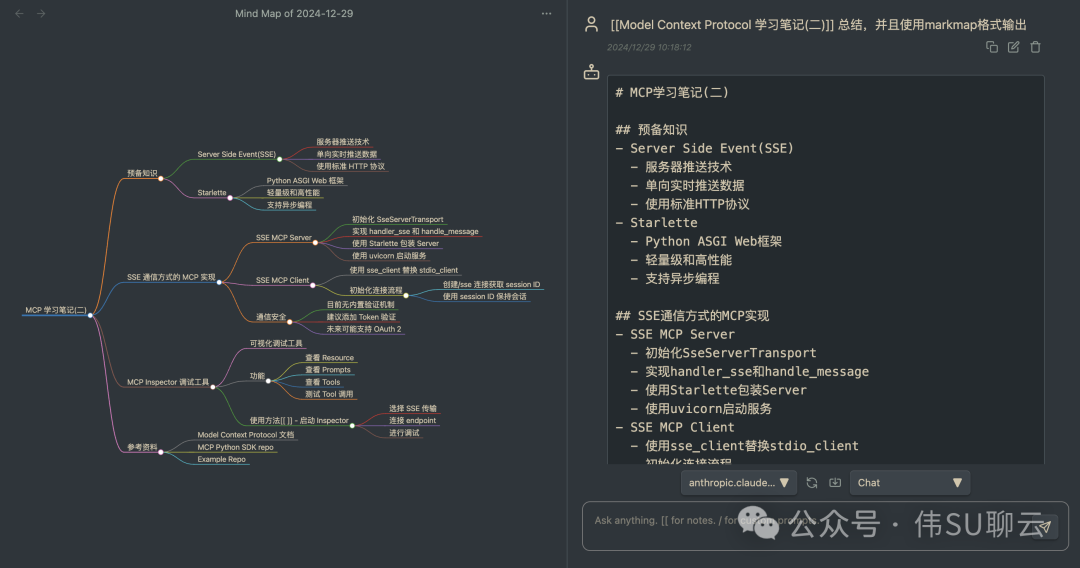
Project reference links, usage
3.
Obsidian-Copilot Code Explanation
Next, let’s take a look at the code of Copilot. Once familiar, you can add more features yourself.
3.1 Model Variables, Initialization Parameters:
First, we need to add the Amazon Bedrock model IDs in src/constants.ts.
export enum ChatModels {
......
AMAZON_BEDROCK_CLAUDE_3_5_SONNET="anthropic.claude-3-5-sonnet-20241022-v2:0",
AMAZON_BEDROCK_CLAUDE_3_5_HAIKU="anthropic.claude-3-5-haiku-20241022-v1:0",
AMAZON_BEDROCK_NOVA_LITE="amazon.nova-lite-v1:0",
AMAZON_BEDROCK_NOVA_PRO="amazon.nova-pro-v1:0",
}
We also need to add the connection parameters for Amazon Bedrock in src/aiParams.ts: Access Key, Secret Access Key, Region.
export interface ModelConfig {
......
amazonBedrockApiKey?: string;
amazonBedrockApiSecretKey?: string;
amazonBedrockRegion?: string;
}
3.2 UI:
Next, we need to extend the plugin configuration interface.
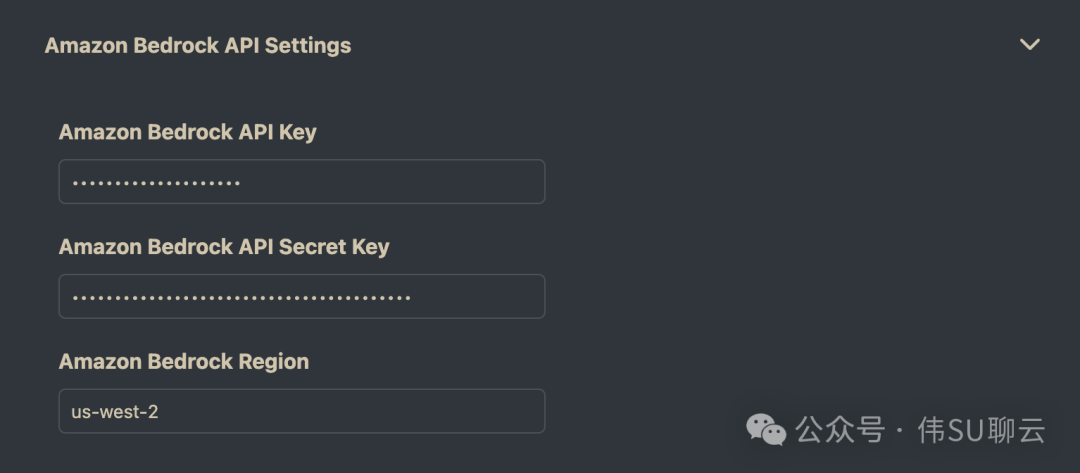
src/settings/components/ApiSettings.tsx
<Collapsible title="Amazon Bedrock API Settings">
<div>
<ApiSetting
title="Amazon Bedrock API Key"
value={amazonBedrockApiKey}
setValue={setAmazonBedrockApiKey}
placeholder="Enter Amazon Bedrock API Key"
/>
<ApiSetting
title="Amazon Bedrock API Secret Key"
value={amazonBedrockApiSecretKey}
setValue={setAmazonBedrockApiSecretKey}
placeholder="Enter Amazon Bedrock API Secret Key"
/>
<ApiSetting
title="Amazon Bedrock Region"
value={amazonBedrockRegion}
setValue={setAmazonBedrockRegion}
placeholder="Enter Amazon Bedrock Region"
type="text"
/>
</div>
</Collapsible>
3.3 Add Amazon Bedrock Inference:
Referring to the original project, we continue to use the @langchain library, modify src/LLMProviders/chatModelManager.ts, and add the ChatBedrockConverse library from @langchain/aws to implement support for Amazon Bedrock. The code mainly modifies the providerConfig, buildModelMap, and setChatModel objects/functions in the ChatModelManager class.
import {ChatBedrockConverse} from '@langchain/aws';
export default class ChatModelManager {
const providerConfig = {
......
[ChatModelProviders.AMAZON_BEDROCK]: {
region: params.amazonBedrockRegion || "us-west-2",
credentials: {
accessKeyId: decrypt(params.amazonBedrockApiKey),
secretAccessKey: decrypt(params.amazonBedrockApiSecretKey),
},
model: customModel.name,
},
}
}
Other code can be found in the commit log of the GitHub repo.
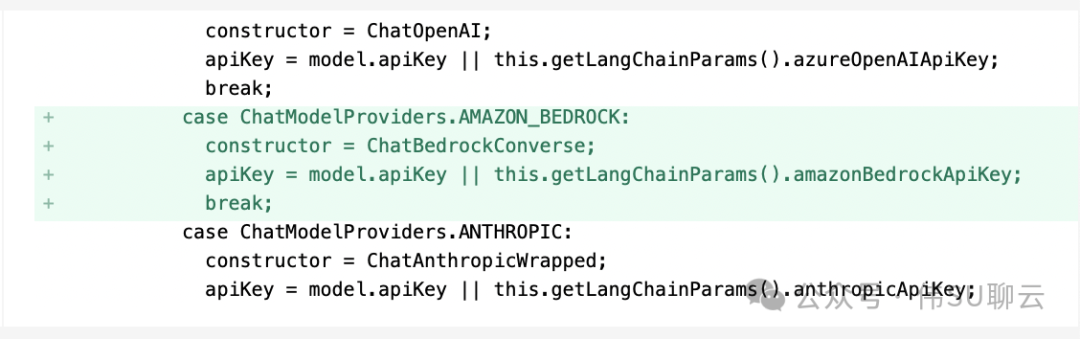
4.
Conclusion
Obsidian is a personal knowledge management software suitable for builders, especially for those who love to tinker. Through TypeScript/JavaScript, it can be extended according to our needs and imagination, such as adding a Model Context Protocol Server interface or a local RAG. Have fun!