1. Define the Problem and Task
Before constructing an agent, it is essential to clarify the agent’s goals and tasks. The definition of the task determines the actions the agent needs to perform and will influence multiple choices in the agent’s design. Consider the following aspects:
- Environment: In which environment will the agent operate? Is the environment open or closed?
- Goal: What is the agent’s goal? For example, maze navigation, enemy confrontation in a game, autonomous driving, etc.
- Rewards and Feedback: How does the agent receive feedback based on the actions taken? For instance, in reinforcement learning, the agent can receive rewards and penalties from the environment.
2. Select Appropriate Algorithms and Models
Choose the appropriate algorithm to control the agent’s behavior based on the characteristics of the task. This typically includes the following approaches:
-
Rule-based Agents (e.g., expert systems, decision trees, etc.):
- Make decisions based on predefined rules derived from the state of the environment. Suitable for scenarios where the problem is clear and rules are easy to define.
-
Reinforcement Learning (RL) Agents:
- Reinforcement learning is one of the mainstream methods for building agents. The agent learns how to act in various states to maximize long-term rewards through interaction with the environment. Common reinforcement learning algorithms include:
- Q-learning is a value iteration method used for discrete state-action spaces.
- Deep Q Network (DQN) combines Q-learning with deep neural networks, suitable for high-dimensional state spaces.
- Policy Gradient directly optimizes the policy to find the optimal strategy, commonly used in complex action spaces.
- Reinforcement learning is one of the mainstream methods for building agents. The agent learns how to act in various states to maximize long-term rewards through interaction with the environment. Common reinforcement learning algorithms include:
-
Deep Learning Models:
- When tasks involve complex input data such as vision and speech recognition, deep neural networks (e.g., Convolutional Neural Networks (CNN), Recurrent Neural Networks (RNN)) can help agents extract features from raw data for decision-making.
-
Evolutionary Algorithms (e.g., genetic algorithms, particle swarm optimization):
- Used to solve optimization problems, especially when there is no explicit model, evolutionary algorithms can gradually improve the agent’s performance.
3. Environment Modeling and Simulation
Environment simulation is the foundation of the agent’s interaction with the world; the environment can be virtual or physical. The environment needs the following characteristics:
- State Space: Defines all possible states in the environment. For example, in a maze problem, the state space is all positions within the maze.
- Action Space: All possible actions the agent can choose. For instance, the agent can choose to “move up,” “move down,” etc.
- Reward Function: The reward or penalty the agent receives after performing an action, usually related to the task’s goal. For example, in reinforcement learning, the agent might receive a reward (or penalty) each time it takes a step.
If in a physical environment (e.g., robotics), environment modeling becomes more complex, potentially involving sensors (e.g., cameras, LiDAR), actuators (e.g., motors, robotic arms), etc.
4. Design the Decision-Making Mechanism of the Agent
The core of the agent is the decision-making mechanism, which determines how the agent makes action decisions from the state of the environment. This includes:
-
Policy: Determines what action the agent takes in each state. The policy can be a simple rule or a complex function (e.g., a deep neural network).
-
Value Function: Evaluates the expected return the agent can achieve in a certain state. Common methods include Q-learning, V(s), etc.
-
Model: Some agents have a model of the environment, predicting changes in the environment and making decisions based on simulations. This method is common in model-based reinforcement learning (Model-based RL).
5. Train the Agent
Training is a crucial part of the agent-building process, where the agent learns how to operate in the environment.
-
Supervised Learning: If you have labeled data (i.e., input and correct output), you can train the agent using supervised learning. A common practice is to train with a large amount of labeled data.
-
Reinforcement Learning: The agent continuously adjusts its policy through interaction with the environment. The training process includes:
- At each step, the agent performs an action.
- Based on the environment’s feedback (reward or penalty), the agent updates its policy or value function.
- This process is optimized through multiple rounds of interaction and repeated training.
Common algorithms for training reinforcement learning agents include:
- Q-learning: Updates the action-value function Q(s,a).
- Deep Q Network (DQN): Approximates the Q function using neural networks.
- Policy Gradient: Directly optimizes the policy, commonly used for more complex tasks.
6. Tuning and Optimization
During the training process of the agent, it may be necessary to adjust parameters to improve performance. These tunings can include:
- Hyperparameter Tuning: Choosing hyperparameters such as learning rate, discount factor, etc.
- Reward Function Design: The design of the reward signal significantly impacts the agent’s learning process. It is essential to ensure that the reward structure guides the agent toward the correct goal.
- Policy Improvement: The policy can be improved through various methods, such as policy iteration, value iteration, etc.
7. Testing and Deployment
The trained agent needs to undergo rigorous testing to ensure it performs stably and meets expectations in various environmental conditions. If the agent can adapt to various environmental changes and maintain effective decision-making, it can be put into practical use.
During actual deployment, some practical issues may arise, such as:
- Hardware Compatibility: If it is a physical robot, hardware compatibility and response speed are critical factors.
- Real-time Performance: The agent needs to make decisions quickly in real-time environments.
- Fault Tolerance: The agent needs to have a certain level of fault tolerance to cope with environmental uncertainties.
8. Continuous Improvement and Maintenance
After the agent is deployed, continuous monitoring and improvement may be necessary. The agent’s capabilities can be enhanced through the following methods:
- Online Learning: The agent can continue to learn and update through new data after deployment.
- Environmental Adaptation: If the environment changes, the agent may need to retrain or adjust its policy to adapt to new situations.
In summary, the process of building an intelligent agent involves several steps, including defining tasks, selecting algorithms, modeling environments, designing decision mechanisms, training, and optimization. Most importantly, it is essential to choose the appropriate technical route based on the specific needs of the problem. In reinforcement learning, agents typically learn and optimize through interaction with the environment, ultimately achieving the desired goals. During actual deployment, attention must also be paid to hardware compatibility, real-time performance, and fault tolerance issues.
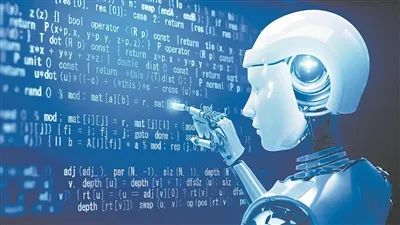
The process of building an intelligent agent can be illustrated with a specific example. We take Reinforcement Learning (RL) as an example to construct an agent to solve a simple task: to find an exit in a maze.
Task Description:
We want to build an agent that can find the exit in a maze environment. The agent can choose to move in four directions: up, down, left, and right. Each move will receive rewards or penalties based on its chosen position, and the ultimate goal is to find the exit and obtain the maximum reward.
Step 1: Define the Problem and Task
-
Environment:
- Assume the maze is a 5×5 grid, and the agent starts from the top left corner of the maze (0,0), aiming to reach the bottom right corner (4,4).
- The state space of the environment consists of all possible positions in the maze, sized 5×5, with a total of 25 states.
-
Action Space:
- The agent’s action space consists of four directions: up (Up), down (Down), left (Left), right (Right).
-
Reward Function:
- When reaching the exit, the agent receives a reward of +10.
- Each move incurs a penalty of -1, encouraging the agent to minimize unnecessary movements.
- If the agent moves outside the maze boundaries, it incurs a penalty (-10).
-
Goal:
- The agent’s goal is to start from (0,0), navigate through several steps to reach the exit (4,4), and minimize detours to achieve the highest total reward.
Step 2: Choose an Algorithm
We will use the Q-learning algorithm, which is a common reinforcement learning algorithm that learns a value function Q(s,a) to represent the expected return for taking a certain action a in a state s.
- Q Function: Q(s,a) stores the expected reward obtained by taking action a in state s.
Step 3: Build the Environment Model
import numpy as np
class MazeEnv:
def __init__(self):
self.size = 5 # Maze size 5x5
self.goal = (4, 4) # Goal position
self.state = (0, 0) # Initial state
def reset(self):
self.state = (0, 0) # Reset state
return self.state
def step(self, action):
x, y = self.state
# Update state based on action
if action == 0: # Up
x = max(0, x - 1)
elif action == 1: # Down
x = min(self.size - 1, x + 1)
elif action == 2: # Left
y = max(0, y - 1)
elif action == 3: # Right
y = min(self.size - 1, y + 1)
self.state = (x, y)
# Check if reached the goal
if self.state == self.goal:
return self.state, 10, True # Reached exit, reward +10
# Check if out of bounds
if x < 0 or x >= self.size or y < 0 or y >= self.size:
return self.state, -10, False # Out of bounds, penalty -10
return self.state, -1, False # Penalty of -1 for each move
Step 4: Implement the Q-learning Algorithm
class QLearningAgent:
def __init__(self, env, alpha=0.1, gamma=0.9, epsilon=0.1):
self.env = env
self.alpha = alpha # Learning rate
self.gamma = gamma # Discount factor
self.epsilon = epsilon # Exploration rate
self.q_table = np.zeros((env.size, env.size, 4)) # Q table: 25 states, each state has 4 actions
def choose_action(self, state):
# epsilon-greedy strategy: randomly choose action with epsilon probability, otherwise choose action with max Q value
if np.random.uniform(0, 1) < self.epsilon:
return np.random.choice(4) # Randomly choose an action
else:
x, y = state
return np.argmax(self.q_table[x, y]) # Choose action with max Q value
def learn(self, state, action, reward, next_state):
x, y = state
nx, ny = next_state
# Q-learning update rule
best_next_action = np.argmax(self.q_table[nx, ny]) # Optimal action for next state
self.q_table[x, y, action] += self.alpha * (reward + self.gamma * self.q_table[nx, ny, best_next_action] - self.q_table[x, y, action])
def train(self, episodes=1000):
for episode in range(episodes):
state = self.env.reset()
done = False
total_reward = 0
while not done:
action = self.choose_action(state)
next_state, reward, done = self.env.step(action)
self.learn(state, action, reward, next_state)
state = next_state
total_reward += reward
if episode % 100 == 0:
print(f"Episode {episode}, Total Reward: {total_reward}")
Step 5: Train the Agent
# Create maze environment and Q-learning agent
env = MazeEnv()
agent = QLearningAgent(env)
# Train the agent
agent.train(episodes=1000)
Step 6: Test the Agent
After training, we can let the agent execute tests in the maze to see if it can find the exit.
# Test the trained agent
state = env.reset()
done = False
while not done:
action = agent.choose_action(state)
next_state, reward, done = env.step(action)
print(f"State: {state}, Action: {action}, Next State: {next_state}, Reward: {reward}")
state = next_state
Results:
Through training, the agent gradually learns how to maximize long-term rewards by continuously trying and updating the Q table, ultimately learning to navigate from the maze’s starting point to the exit.
In this example, we built a reinforcement learning agent through the following steps:
- Define the Task: Allow the agent to navigate from the start to the exit in the maze.
- Select the Algorithm: Use the Q-learning algorithm.
- Build the Environment: Define the maze environment and reward mechanism.
- Train the Agent: Through interaction with the environment, train the agent multiple times to learn how to select the best movement strategy.
- Test the Agent: Use the trained agent to test in the maze and observe whether it can successfully find the exit.
This process demonstrates how to build a simple agent through reinforcement learning. Of course, in practical applications, the environment and tasks will be more complex, and the training of the agent will be more refined.