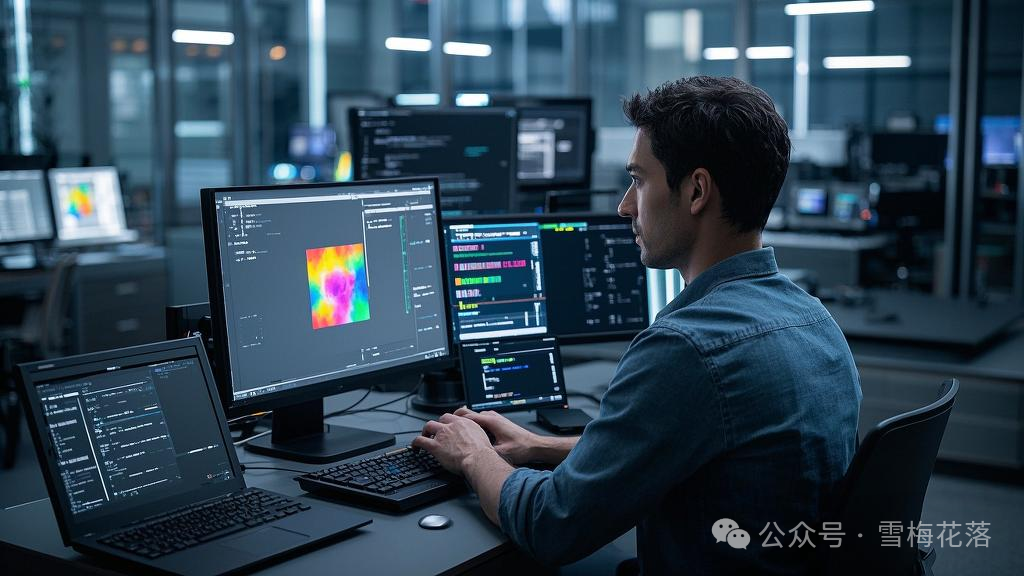
Computer Vision is an important branch of artificial intelligence, and OpenCV (Open Source Computer Vision Library) is one of the most popular open-source libraries in the field of computer vision. It provides a rich set of image processing and computer vision algorithms, widely used in facial recognition, object detection, image segmentation, and more. This article will guide you through the basics of OpenCV and help you master fundamental image recognition techniques through practice.
Installing OpenCV
Before we begin, we need to install the OpenCV library. If you are using Python, you can install it using the following command:
pip install opencv-python
If you also need to use OpenCV’s extension modules (such as SIFT, SURF, etc.), you can install opencv-contrib-python
:
pip install opencv-contrib-python
Reading and Displaying Images
First, let’s start with the most basic operations: reading and displaying images. Below is a simple Python code example:
import cv2
# Read an image
image = cv2.imread('example.jpg')
# Display the image
cv2.imshow('Image', image)
# Wait for a key press
cv2.waitKey(0)
# Close all windows
cv2.destroyAllWindows()
In this example, the cv2.imread()
function is used to read an image file, and the cv2.imshow()
function is used to display the image. The cv2.waitKey(0)
function waits for the user to press any key before closing the window.
Basic Image Operations
Grayscale Conversion
In image processing, converting a color image to a grayscale image is a common operation. Grayscale images have only one channel and are more efficient to process. The following code demonstrates how to convert a color image to a grayscale image:
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Image Resizing
Image resizing is another common operation. We can use the cv2.resize()
function to adjust the size of the image:
resized_image = cv2.resize(image, (300, 200)) # Resize the image to 300x200 pixels
cv2.imshow('Resized Image', resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Image Recognition: Edge Detection
Edge detection is an important step in image recognition, commonly used in object detection and image segmentation. OpenCV offers various edge detection algorithms, with the most commonly used being the Canny edge detector. Below is a simple Canny edge detection example:
edges = cv2.Canny(gray_image, 100, 200) # Use Canny algorithm to detect edges
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, the cv2.Canny()
function takes three parameters: the input image, the low threshold, and the high threshold. By adjusting these two thresholds, you can control the number of detected edges.
Face Recognition
Face recognition is a popular application in computer vision. OpenCV provides a pre-trained face detection model that we can use directly to detect faces in images. Below is a simple face detection example:
# Load the pre-trained face detection model
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# Detect faces
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# Mark detected faces in the image
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
# Display the result
cv2.imshow('Detected Faces', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, the cv2.CascadeClassifier()
is used to load the pre-trained face detection model, and the detectMultiScale()
function is used to detect faces in the image. Detected faces are marked with rectangles.
Conclusion
Through this article, you have learned the basic operations of OpenCV, including reading, displaying, grayscale conversion, resizing, edge detection, and face recognition. These techniques are the foundation of computer vision, and mastering them will lay a solid foundation for your further exploration in image processing and recognition.
We hope this article helps you quickly get started with OpenCV and inspires your interest in computer vision. If you have any questions or suggestions, feel free to leave a comment for discussion.
#OpenCV #ComputerVision #ImageRecognition