NLTK: The Swiss Army Knife for Natural Language Processing
Natural Language Processing has always been a hot topic in the field of AI, but to be honest, it can be quite challenging without the right tools. I remember when I first started with NLP, just the text preprocessing took me a long time. However, once I encountered NLTK, my whole life changed. It’s like a toolbox filled with various small tools—want to tokenize? Want part-of-speech tagging? Want sentiment analysis? It’s all there, and the best part is that it’s incredibly easy to use.
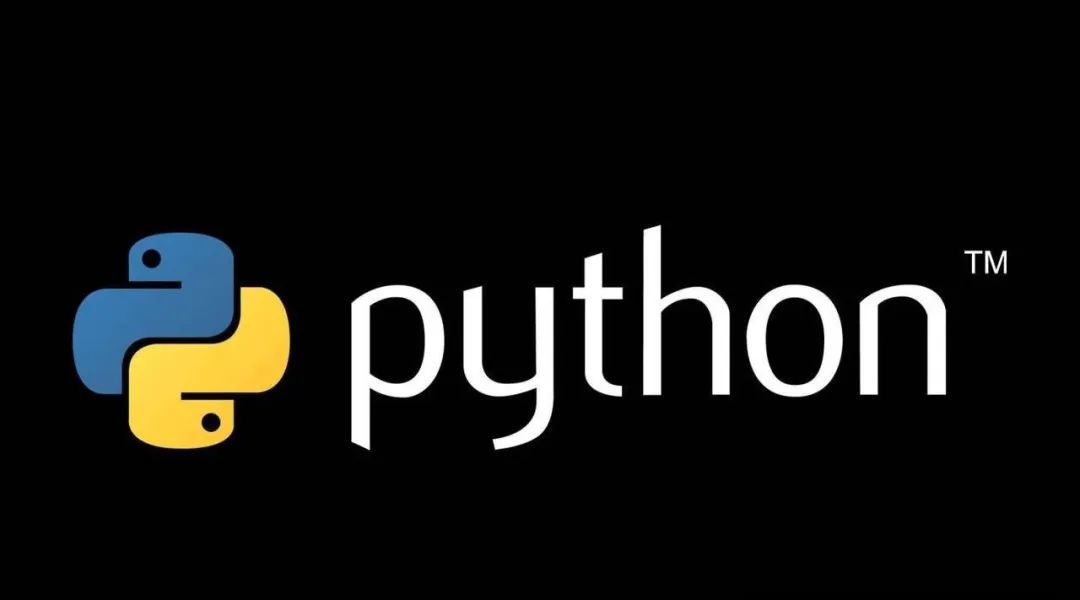
1.
Installation and Setup
# Install NLTK
pip install nltk
# Download necessary packages
import nltk
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
Tip: If downloading the packages is particularly slow, it is recommended to switch to a domestic mirror source or directly download the packages manually from the NLTK website.
2.
Tokenization Techniques
Tokenization is probably the most fundamental operation in text processing, and the tokenizer in NLTK is incredibly useful:
from nltk.tokenize import word_tokenize, sent_tokenize
text = "I have a puppy that loves to eat bones. Every morning, it pesters me for food!"
sentences = sent_tokenize(text) # Sentence tokenization
words = word_tokenize(text) # Word tokenization
print(f"Sentence tokenization result: {sentences}")
print(f"Word tokenization result: {words}")
Tokenization may seem simple, but there is a lot of knowledge behind it. The strategies for tokenizing Chinese and English are completely different; English relies on spaces, while Chinese relies on algorithms, which showcases the power of NLTK.
3.
Part-of-Speech Tagging Tricks
Part-of-speech tagging is about determining whether each word is a noun, verb, or adjective. It sounds simple, but it’s not that easy:
from nltk import pos_tag
from nltk.tokenize import word_tokenize
text = "The quick brown fox jumps over the lazy dog"
tokens = word_tokenize(text)
tagged = pos_tag(tokens)
print(tagged)
Tip: The pos_tag function is primarily designed for English; for Chinese part-of-speech tagging, you need to use other modules like jieba.
4.
Effortless Word Frequency Counting
Want to know which words appear most frequently in an article? FreqDist is designed for that:
from nltk.probability import FreqDist
words = ['python', 'is', 'awesome', 'python', 'rocks']
fdist = FreqDist(words)
print(fdist.most_common(3)) # Display the 3 most common words
5.
Sentiment Analysis Made Easy
NLTK can also analyze the sentiment of the text, determining whether it is positive or negative:
from nltk.sentiment import SentimentIntensityAnalyzer
sia = SentimentIntensityAnalyzer()
text = "I love this movie! It's amazing!"
print(sia.polarity_scores(text))
Tip: The accuracy of sentiment analysis is highly dependent on the quality of the training data, so don’t expect it to understand overly complex emotional expressions.
Key Takeaways:
-
NLTK supports a wide range of language processing features, so just learn what you need.
-
Be sure to master basic functions like tokenization and part-of-speech tagging.
-
When processing Chinese, remember to consider encoding issues.
-
Data preprocessing is crucial; garbage in, garbage out.
-
Refer to the official documentation frequently; it contains many example codes.
When coding, remember to handle exceptions to avoid program crashes. Consider performance issues when dealing with large datasets, and use generators when necessary.
If you want to master NLTK, besides having a solid foundation in Python, you should also have a basic understanding of linguistic concepts. But don’t worry, take your time, practice more, and you can become an NLP expert too.
Disclaimer: This story is purely fictional, and any resemblance to actual persons, events, or institutions is purely coincidental and not intentional.
If you like this article, please give it a thumbs up and share!