In modern corporate decision-making processes, it is often necessary to address issues of fuzziness and uncertainty. This article will introduce how to build an expert system based on fuzzy reasoning in Excel to achieve intelligent decision support.
Application Scenarios
– Credit risk assessment
– Product quality control
– Talent recruitment screening
– Portfolio optimization
– Supplier rating
Implementation Steps
1. Define Fuzzy Sets
“`excel
=LAMBDA(x, a, b, c,<br/> IF(x <= a, 0,<br/> IF(x <= b, (x-a)/(b-a),<br/> IF(x <= c, (c-x)/(c-b), 0))))<br/>(E2, A2, B2, C2)<br/>
“`
Parameter Explanation:
– x: Input value
– a: Left endpoint
– b: Peak point
– c: Right endpoint
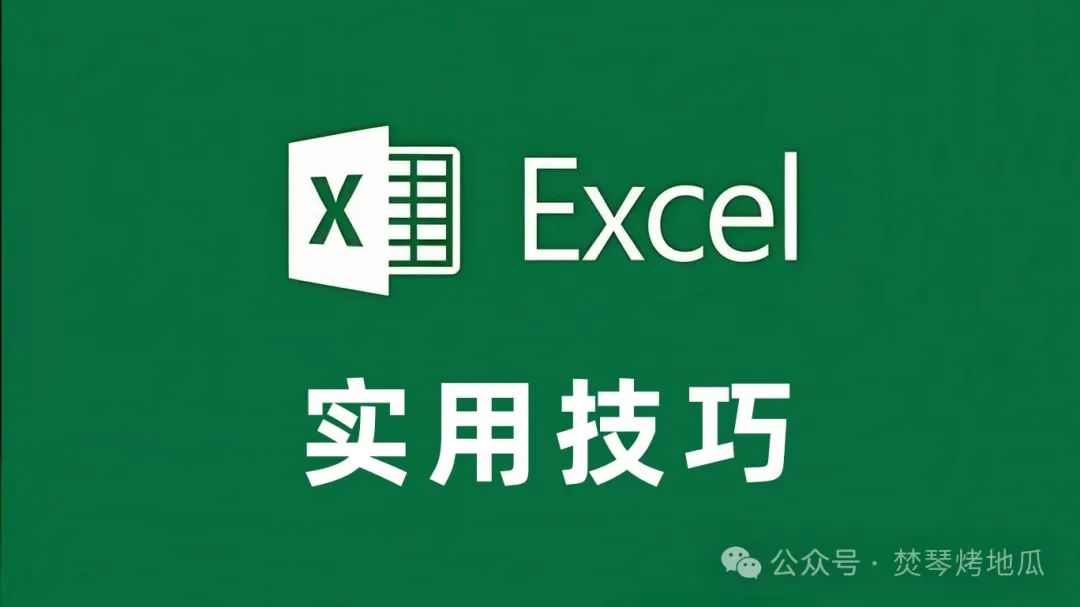
2. Build Fuzzy Rule Base
“`vba
Public Sub BuildFuzzyRulebase()<br/> Const MAX_RULES As Integer = 100<br/> <br/> 'Initialize rule structure<br/> Type FuzzyRule<br/> InputVars() As Double<br/> OutputVar As Double<br/> Weight As Double<br/> End Type<br/> <br/> 'Create rules array<br/> Dim rules() As FuzzyRule<br/> ReDim rules(1 To MAX_RULES)<br/> <br/> 'Load rules from worksheet<br/> Dim ruleSheet As Worksheet<br/> Set ruleSheet = ThisWorkbook.Sheets("Rules")<br/> <br/> For i = 1 To MAX_RULES<br/> With rules(i)<br/> .InputVars = ruleSheet.Range("B" & i + 1).Value<br/> .OutputVar = ruleSheet.Range("C" & i + 1).Value<br/> .Weight = ruleSheet.Range("D" & i + 1).Value<br/> End With<br/> Next i<br/>End Sub<br/>
“`
3. Fuzzy Inference Engine
“`vba
Private Function FuzzyInference(ByRef inputs() As Double) As Double<br/> 'Define membership functions<br/> Dim membershipValues() As Double<br/> ReDim membershipValues(1 To UBound(inputs))<br/> <br/> 'Calculate membership degrees<br/> For i = 1 To UBound(inputs)<br/> membershipValues(i) = CalculateMembership(inputs(i))<br/> Next i<br/> <br/> 'Apply inference rules<br/> Dim inferenceResult As Double<br/> inferenceResult = ApplyRules(membershipValues)<br/> <br/> 'Defuzzification<br/> FuzzyInference = Defuzzify(inferenceResult)<br/>End Function<br/>
“`
4. Membership Degree Calculation
“`excel
=LAMBDA(input_value, membership_params,<br/> LET(<br/> type, INDEX(membership_params, 1),<br/> params, INDEX(membership_params, 2):INDEX(membership_params, 4),<br/> SWITCH(type,<br/> 1, Triangular(input_value, params),<br/> 2, Gaussian(input_value, params),<br/> 3, Trapezoidal(input_value, params),<br/> "Invalid type"<br/> )<br/> )<br/>)(A2, B2:E2)<br/>
“`
5. Defuzzification
“`vba
Private Function Defuzzify(ByRef fuzzySet() As Double) As Double<br/> 'Center of gravity method<br/> Dim numerator As Double, denominator As Double<br/> <br/> For i = 1 To UBound(fuzzySet)<br/> numerator = numerator + (i * fuzzySet(i))<br/> denominator = denominator + fuzzySet(i)<br/> Next i<br/> <br/> If denominator <> 0 Then<br/> Defuzzify = numerator / denominator<br/> Else<br/> Defuzzify = 0<br/> End If<br/>End Function<br/>
“`
Advanced Optimization Techniques
1. Dynamic Adjustment of Rule Weights
“`vba
Private Sub UpdateRuleWeights(ByRef rules() As FuzzyRule)<br/> 'Calculate rule performance<br/> Dim performance() As Double<br/> ReDim performance(1 To UBound(rules))<br/> <br/> For i = 1 To UBound(rules)<br/> performance(i) = CalculateRulePerformance(rules(i))<br/> <br/> 'Update weights based on performance<br/> rules(i).Weight = rules(i).Weight * (1 + performance(i))<br/> Next i<br/> <br/> 'Normalize weights<br/> Call NormalizeWeights(rules)<br/>End Sub<br/>
“`
2. Fuzzy Set Optimization
“`excel
=LAMBDA(data, num_clusters,<br/> LET(<br/> centers, SEQUENCE(num_clusters),<br/> distances, MMULT(data-centers, TRANSPOSE(data-centers)),<br/> memberships, 1/(1 + distances^2),<br/> normalized, memberships/SUM(memberships),<br/> normalized<br/> )<br/>)(A2:A100, 3)<br/>
“`
Usage Notes
– Input Range: Ensure input values are within the defined domain
– Number of Rules: It is recommended not to exceed 200 rules
– Computational Efficiency: Consider batch processing for large-scale calculations
– Memory Management: Clean up large arrays in a timely manner
– Numerical Precision: Be cautious of floating-point calculation errors
Shortcut Tips:
– Alt+F11: Open VBA Editor
– F5: Run Code
– Ctrl+Shift+Enter: Array Formula Calculation
– Alt+I+R: Insert Reference
– Ctrl+G: Go to Specific Cell
This system formalizes expert experiences through fuzzy logic and is suitable for various decision support scenarios. It is recommended to adjust fuzzy rules and membership functions based on actual business characteristics, and machine learning methods can be introduced to optimize parameters if necessary. For real-time decision scenarios, it is recommended to implement a rule caching mechanism to improve response speed.