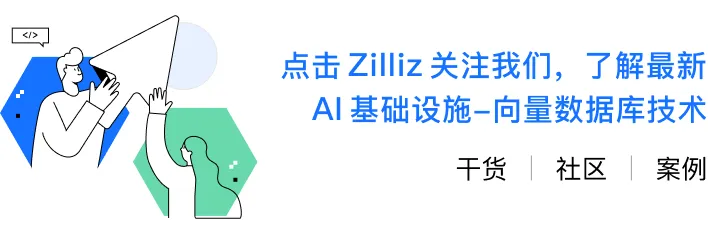
How to Build a Face Recognition Application from Scratch? Try the combination of native Java AI algorithm: EasyAi + Milvus.
The software and tools used in this article include:
-
EasyAi: Face feature vector extraction
-
Milvus: Vector database for efficient storage and retrieval of data.
01.
EasyAi: The Most Popular Java AI Algorithm Framework in China
As a framework for developing AI applications in pure Java, EasyAi has no dependencies. It is a native Java AI algorithm framework. First, it can be smoothly integrated into our Java project with Maven, requiring no additional environment configuration or dependencies, making it ready to use out of the box. Moreover, it has some pre-packaged modules for image object detection and AI customer service, and provides various tools for deep learning, machine learning, reinforcement learning, heuristic learning, matrix operations, derivative functions, partial derivative functions, and other low-level algorithms. Developers can complete the development of small micro-models tailored to their business through simple learning.
02.
EasyAi-Face: Face Recognition Application Based on Easy-Ai
1. Generate an Average Human Face, by scaling all face samples to a uniform size, truncating excess top and bottom, padding with zeros where necessary, summing all pixel channels to obtain the average, and outputting the average face.
2. Use the pre-trained face localization fastYolo model to locate the target photo and set a threshold, so that only faces with confidence exceeding this threshold are considered.
3. Obtain the face localization box with the highest confidence in the target photo and conduct a secondary correction based on this localization.
Secondary Correction Plan:
-
Using particle swarm optimization, set four feature dimensions to seek the optimal solution, which are the x and y coordinates of the top left corner of the face and its width and height. The adaptive function return value is set to the minimum value optimal. The xy coordinates and width and height of the four-dimensional particles adjust their activity range, with upper and lower limits set to the +/-50 pixel range of the initial localization coordinates and dimensions (adjustable).
-
The adaptive function calculation process involves cropping the face based on the coordinates locked by the four-dimensional particles, resizing it according to the previous scaling plan to a specified smaller size, and applying softMax to the grayscale channels to probabilistically convert all values in the matrix.
-
Compare the average face with the Euclidean distance of the particle-locked face grayscale probability image at this point, and return. Allow particles to explore (within the specified number of iterations) for the minimum value optimal solution.
4. Obtain Face Features, by capturing the image based on the optimal coordinates found by the particles, and cropping the image from the top to 70% of its height (discarding the mouth, as its stability is relatively poor), to extract the LBP local binary texture features of the image.
03.
Building a Face Recognition Application with EasyAi-Face + Milvus
3.1 Extracting Face Features
Import Dependencies
<dependency>
<groupId>org.dromara.easyai</groupId>
<artifactId>seeFace</artifactId>
<version>1.0.5</version>
</dependency>
Initialize Face
@Bean
public Face face(FaceConfig faceConfig ){
if (StringUtils.isNotBlank(faceConfig.getAvgFace()) && StringUtils.isNotBlank(faceConfig.getFaceModel())) {
return FaceFactory.getFace(faceConfig.getAvgFace(), faceConfig.getFaceModel());
}
return FaceFactory.getFace();
}
Extract Face Features
private List<Float> getFloats(InputStream inputStream) {
ThreeChannelMatrix m = Picture.getThreeMatrix(inputStream, false);
ErrorMessage errorMessage = face.look(m, idWorker.nextId(), 30);
final Matrix feature = errorMessage.getFaceMessage().getFeature();
return MatrixUtil.matrixToFloatList(feature);
}
3.2 Store in Vector Database
public void initUserVector(UserDTO userDTO, List<Float> features) {
List<String> names = Collections.singletonList(userDTO.getUserName());
List<Long> userIds = Collections.singletonList(userDTO.getUserId());
List<String> getFaceUrl = Collections.singletonList(userDTO.getFaceUrl());
List<String> getFaceFeatureUrl = Collections.singletonList(userDTO.getFaceFeatureUrl());
List<List<Float>> vectors = Collections.singletonList(features);
List<Field> fields = new ArrayList();
fields.add(new Field("vector", vectors));
fields.add(new Field("face_url", getFaceUrl));
fields.add(new Field("face_feature_url", getFaceFeatureUrl));
fields.add(new Field("user_id", userIds));
fields.add(new Field("user_name", names));
InsertParam insertParam = InsertParam.newBuilder().withCollectionName(milvusConfig.getCollectionName()).withFields(fields).build();
this.milvusClient.insert(insertParam);
}
3.3 Face Recognition: L2 Similarity Search
public List<UserDTO> search(List<Float> floatList, Integer topK) {
final List<SearchResultsWrapper.IDScore> idScoreList = vectorService.search(floatList, topK);
List<UserDTO> list = new ArrayList<>();
idScoreList.forEach(idScore -> {
UserDTO imageDTO = new UserDTO();
final float score = idScore.getScore();
final Map<String, Object> fieldValues = idScore.getFieldValues();
imageDTO.setAutoId(Long.valueOf(String.valueOf(fieldValues.getOrDefault("Auto_id", "-1"))));
imageDTO.setUserId(Long.valueOf(String.valueOf(fieldValues.getOrDefault("user_id", "-1"))));
imageDTO.setUserName(String.valueOf((fieldValues.getOrDefault("user_name", ""))));
imageDTO.setFaceUrl(String.valueOf((fieldValues.getOrDefault("face_url", ""))));
imageDTO.setFaceFeatureUrl(String.valueOf((fieldValues.getOrDefault("face_feature_url", ""))));
imageDTO.setScore(Math.sqrt(score));
list.add(imageDTO);
});
return list;
}
04.
Conclusion
This article demonstrates how to build a face recognition application using EasyAi and Milvus. By combining the advantages of the EasyAi Java ecosystem and Milvus vector search, we can quickly create our own face recognition projects in Java. We hope this article is helpful to you. At the same time, we encourage you to use EasyAi and vector search in your projects to explore more possibilities. The code involved in this article can be obtained from Gitee: Easy-Ai-Face(https://gitee.com/fushoujiang/easy-ai-face).
Recommended Reading
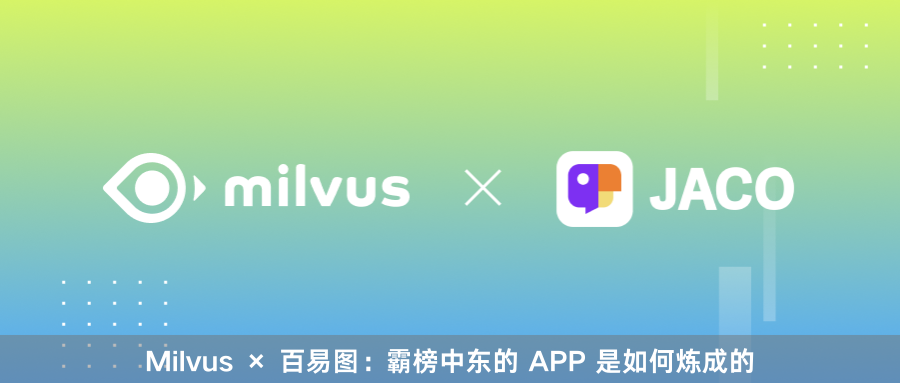
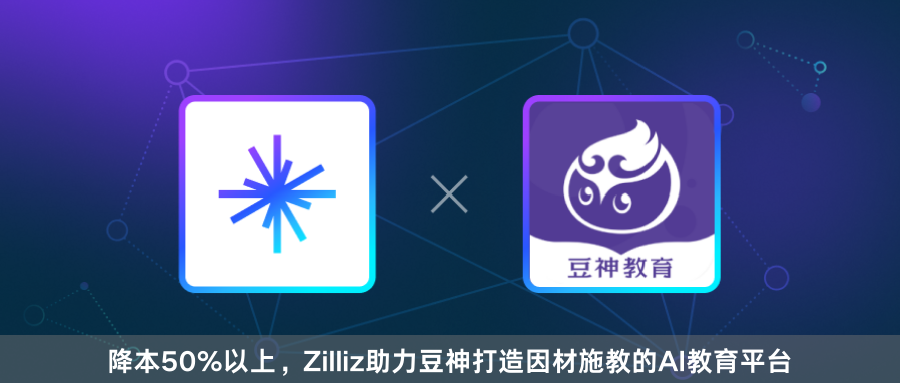
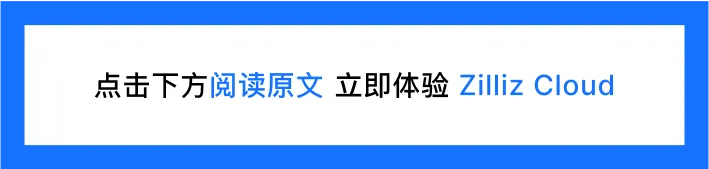