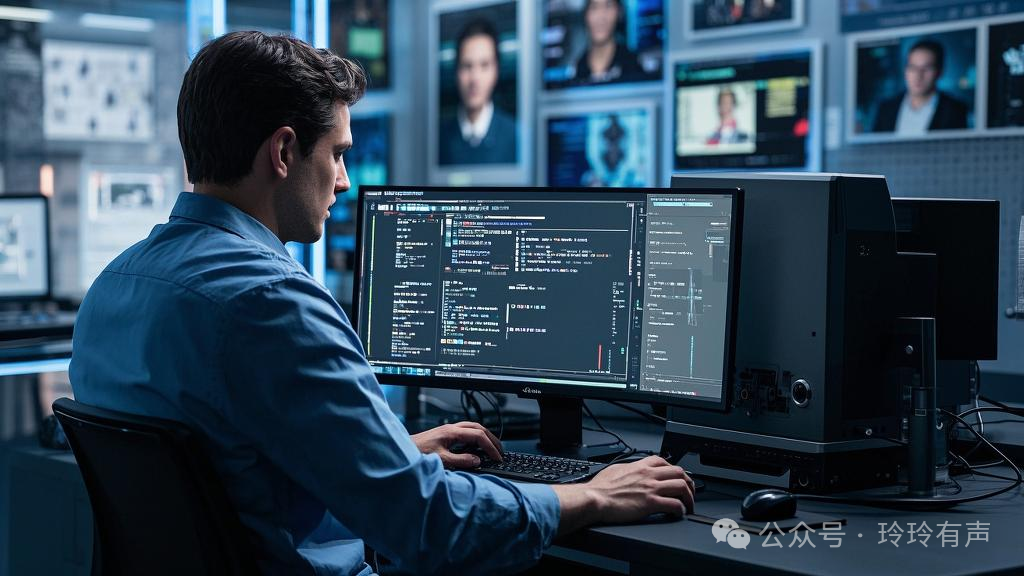
Introduction to OpenCV
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It contains hundreds of computer vision algorithms and is widely used in areas such as image processing, video analysis, object detection, and face recognition. OpenCV supports multiple programming languages, including C++, Python, and Java, and can run on various platforms such as Windows, Linux, and macOS.
Installing OpenCV
Before using OpenCV, you need to install it. Here are the steps to install OpenCV in a Python environment:
pip install opencv-python
If you also need to use additional modules of OpenCV (such as opencv_contrib
), you can use the following command:
pip install opencv-python opencv-contrib-python
Reading and Displaying Images
One of the basic functionalities of OpenCV is reading and displaying images. Here is a simple example that demonstrates how to read and display an image using OpenCV:
import cv2
# Read the image
image = cv2.imread('example.jpg')
# Display the image
cv2.imshow('Image', image)
# Wait for a key press
cv2.waitKey(0)
# Close all windows
cv2.destroyAllWindows()
Code Explanation
-
cv2.imread('example.jpg')
: Reads the image file namedexample.jpg
. -
cv2.imshow('Image', image)
: Displays the image in a window namedImage
. -
cv2.waitKey(0)
: Waits for the user to press any key. The parameter0
means wait indefinitely. -
cv2.destroyAllWindows()
: Closes all open windows.
Basic Image Processing
Grayscale Conversion
Converting a color image to a grayscale image is a common operation in image processing. OpenCV provides the cv2.cvtColor()
function to achieve this:
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Image Resizing
Image resizing is the operation of adjusting the size of an image. OpenCV provides the cv2.resize()
function to perform image resizing:
resized_image = cv2.resize(image, (300, 200)) # Resize image to 300x200 pixels
cv2.imshow('Resized Image', resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Image Rotation
Image rotation can be achieved using the cv2.getRotationMatrix2D()
and cv2.warpAffine()
functions:
(h, w) = image.shape[:2]
center = (w // 2, h // 2)
matrix = cv2.getRotationMatrix2D(center, 45, 1.0) # Rotate 45 degrees
rotated_image = cv2.warpAffine(image, matrix, (w, h))
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Image Recognition Practice
Edge Detection
Edge detection is an important step in image recognition, and a commonly used algorithm is the Canny edge detector. OpenCV provides the cv2.Canny()
function to implement this:
edges = cv2.Canny(gray_image, 100, 200)
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
Face Detection
OpenCV has a built-in Haar cascade classifier that can be used for face detection. Here is a simple example:
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5)
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow('Detected Faces', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Code Explanation
-
cv2.CascadeClassifier()
: Loads the Haar cascade classifier. -
detectMultiScale()
: Detects faces in the image and returns the rectangular coordinates of the faces. -
cv2.rectangle()
: Draws a rectangle on the image to mark the detected faces.
Conclusion
This article introduced the basics of OpenCV, including reading, displaying, grayscale conversion, resizing, and rotating images, as well as image recognition techniques such as edge detection and face detection. With this foundational knowledge and practice, you can further explore the powerful features of OpenCV and apply them to more complex computer vision tasks.
#OpenCV #ImageProcessing #FaceDetection